Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial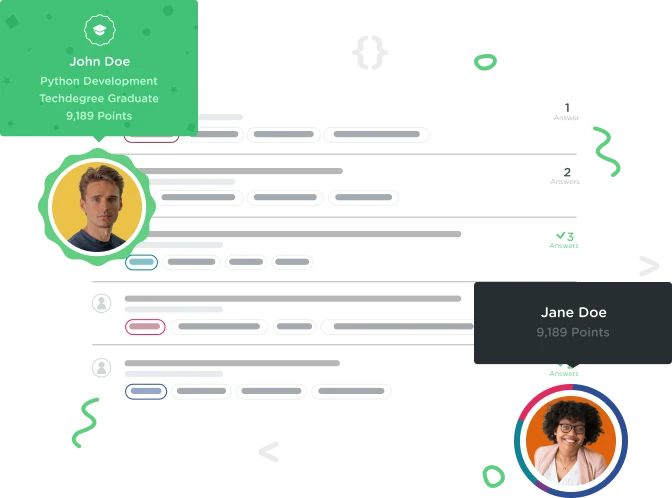

heather wolfram
1,594 PointsWhat is wrong with my code?
I have already requested help with this code multiple times and nothing has worked so far. I am just about crazy from thinking! What am I doing wrong? Is there possibly something wrong with Treehouse that is not allowing for my code to go through??
class Point {
var x: Int
var y: Int
init(x: Int, y: Int) {
self.x = x
self.y = y
}
}
class Machine {
var location: Point
init() {
self.location = Point(x: 0, y: 0)
}
func move(_ direction: String) {
print("Do nothing! Im a machine!")
}
}
// Enter your code below
override func move(_ direction: String) {
switch direction {
case "Up":
location.y += 1
case "Down":
location.y -= 1
case "Right":
location.x += 1
case "Left":
location.x -= 1
default:
break
}
}
1 Answer

andren
28,558 PointsIt's not an issue with the challenge, the instructions for this task specify:
Your task is to subclass Machine and create a new class named Robot. In the Robot class, override the move method and provide the following implementation.
You have done the second part of that but not the first, an overridden method can't exist in a vacuum. It needs to be inside a class which inherits from a class that has the same method already defined. If that is not the case there wouldn't be a point to overriding it in the first place.
If you make a Robot class that inherits from the Machine class and paste your code into it like this:
class Point {
var x: Int
var y: Int
init(x: Int, y: Int) {
self.x = x
self.y = y
}
}
class Machine {
var location: Point
init() {
self.location = Point(x: 0, y: 0)
}
func move(_ direction: String) {
print("Do nothing! I'm a machine!")
}
}
// Enter your code below
class Robot: Machine { // Create a class that inherit from the Machine class.
override func move(_ direction: String) {
switch direction {
case "Up":
location.y += 1
case "Down":
location.y -= 1
case "Right":
location.x += 1
case "Left":
location.x -= 1
default:
break
}
}
}
Then your code will pass.