Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial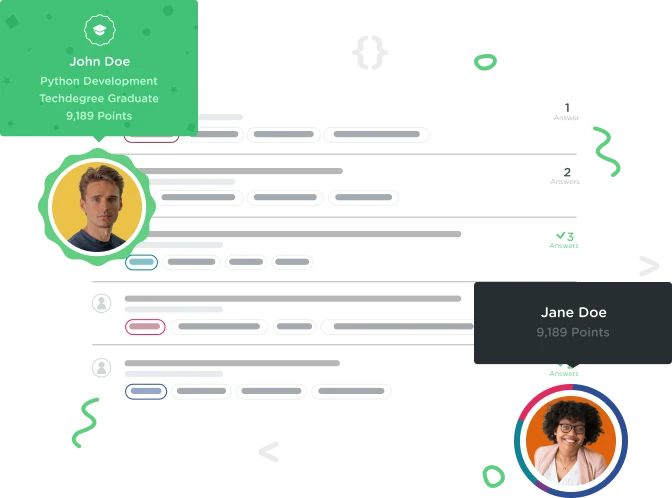

Moe Shanti
519 PointsWhat is wrong with my code
Hi, the message i am given when i run this code: Illegal argument Exeception"invalid..." look around line 15
public class Order {
private String itemName;
private int priceInCents;
private String discountCode;
public Order(String itemName, int priceInCents) {
this.itemName = itemName;
this.priceInCents = priceInCents;
}
private String normalizeDiscountCode (String discountCode){
for( char c : discountCode.toCharArray())
{
if (!Character.isLetter(c) || c != '$'){
throw new IllegalArgumentException ("Invalid discount code");
}
}
discountCode = discountCode.toUpperCase();
return discountCode;
}
public String getItemName() {
return itemName;
}
public int getPriceInCents() {
return priceInCents;
}
public String getDiscountCode() {
return discountCode;
}
public void applyDiscountCode(String discountCode) {
discountCode = normalizeDiscountCode(discountCode);
this.discountCode = discountCode;
}
}
public class Example {
public static void main(String[] args) {
// This is here just for example use cases.
Order order = new Order(
"Yoda PEZ Dispenser",
600);
// These are valid. They are letters and the $ character only
order.applyDiscountCode("abc");
order.getDiscountCode(); // ABC
order.applyDiscountCode("$ale");
order.getDiscountCode(); // $ALE]
try {
// This will throw an exception because it contains numbers
order.applyDiscountCode("ABC123");
} catch (IllegalArgumentException iae) {
System.out.println(iae.getMessage()); // Prints "Invalid discount code"
}
try {
// This will throw as well, because it contains a symbol.
order.applyDiscountCode("w@w");
}catch (IllegalArgumentException iae) {
System.out.println(iae.getMessage()); // Prints "Invalid discount code"
}
}
}
1 Answer

Graham Huelscher
7,003 PointsHi Moe,
The issue lies with your if statement here:
if (!Character.isLetter(c) || c != '$'){ throw new IllegalArgumentException ("Invalid discount code");
Since you are using the OR operator, when the character variable "c" is not the '$', the if statement evaluates to true and the exception is thrown.
For example: c = a.
We'll look at the overall if statement piece by piece
if (!Character.isLetter(c) || c != '$')
!Character.isLetter(c) => false, since 'a' is a letter.
c != '$' => true, since 'a' != '$'.
Therefore the if statement is:
if(false || true)
which proceeds into the if statement code and the exception is thrown.
Hope that helped!