Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial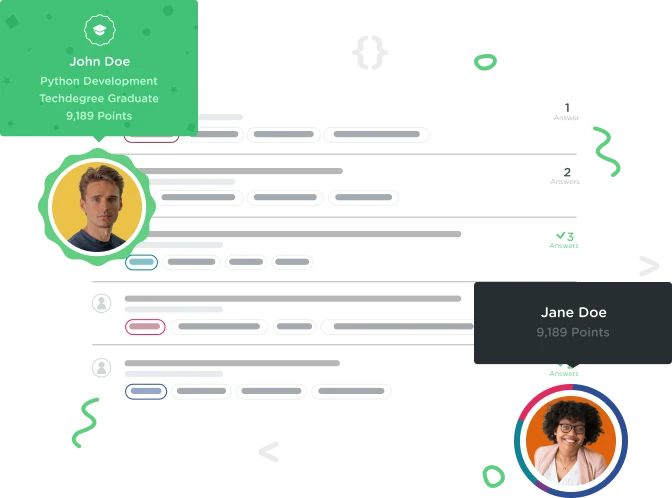

Fábio Nascimento
10,418 PointsWhat is wrong with my code?
Why is this not passing?
var expect = require('chai').expect
describe('subtraction', function () {
var subtraction = require('../WHEREVER')
it('only works with numbers', function () {
// YOUR CODE HERE
expect(subtraction('a', 'b').to.throw('subtraction only works with numbers!');
})
})
function subtraction (number1, number2) {
if (typeof number1 !== 'number' || typeof number2 !== 'number') {
throw Error('subtraction only works with numbers!')
}
return number1 - number2
}
1 Answer
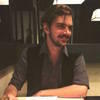
Marco Amadio
4,882 PointsThe problem may be that you're trying to call the "subtraction" function in your code indeed. What you need is to pass the "subtraction" function into an anonymous function, like this:
expect(function () { subtraction('a', 'b') }).to.throw('subtraction only works with numbers!');
Also, you can use function.bind:
expect(subtraction.bind(null, 'a', 'b')).to.throw('subtraction only works with numbers!');
Where the first argument is passed as "this" parameter and other arguments are the function's parameter.
Last but not least, if your function have no parameters, your code will be something like:
/* Your function */
var fn = function () {
throw Error('subtraction only works with numbers!');
}
/* Then in your test code */
expect(fn).to.throw('subtraction only works with numbers!');
Hope it helps.

Fábio Nascimento
10,418 PointsThanks. That helped.

Joshua Kelly
18,830 PointsThis worked! Thanks. I was having problems with Chai. I didn't realize I needed to wrap "subtraction('dog', 'cat')" in an anonymous function to get it to work due to it having parameters.
Ante Adamović
Front End Web Development Techdegree Student 2,508 PointsAnte Adamović
Front End Web Development Techdegree Student 2,508 PointsI'm not an expert but if the code is correct it's not suppose to pass. If the output is 'subtraction only works with numbers!' then the code is doing what it's suppose to ^_^