Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial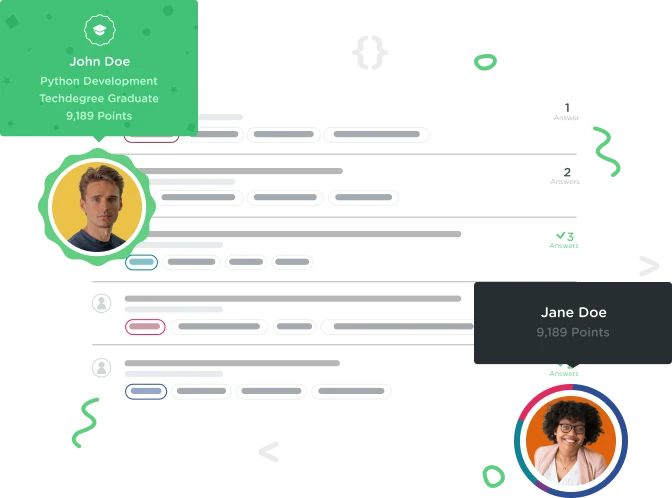
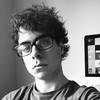
Zach Allan
19,452 PointsWhat is wrong with my code for 'The Conditional Challenge'?
Every time I run this program, the alert tells me the right amount of correctAnswers there are, but it runs the "You know me extremely well. You get a GOLD medal!" line no matter what. What am I doing wrong?
var correctGuess = false;
var correctAnswers = 0;
var favoriteColor = 'blue';
var favoriteAnimal = 'penguin';
var favoriteSport = 'volleyball';
var favoriteMusic = 'indie';
var favoriteFood = 'pizza';
alert("How well do you know me? Answer the questions to find out.");
var question1 = prompt('What is my favorite color?');
if (question1 === favoriteColor) {
correctGuess = true;
correctAnswers += 1;
}
var question2 = prompt('What is my favorite animal?');
if (question2 === favoriteAnimal) {
correctGuess = true;
correctAnswers += 1;
}
var question3 = prompt('What is my favorite sport?');
if (question3 === favoriteSport) {
correctGuess = true;
correctAnswers += 1;
}
var question4 = prompt('What is my favorite genre of music?');
if (question4 === favoriteMusic) {
correctGuess = true;
correctAnswers += 1;
}
var question5 = prompt('What is my favorite food?');
if (question5 === favoriteFood) {
correctGuess = true;
correctAnswers += 1;
}
alert('You got ' + correctAnswers + ' questions correct. Click "OK" to see your ranking.');
if (correctAnswers = 5) {
alert('You know me extremely well. You get a GOLD medal!');
} else if (correctAnswers < 5) {
alert('You know me pretty well. You get a SILVER medal!');
} else if (correctAnswers < 3 && correctAnswers > 0) {
alert("You don't know me very well. You get a BRONZE medal!");
} else {
alert("You don't know me at all. You lose. You receive no prizes");
}
2 Answers
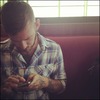
Erik McClintock
45,783 PointsZack,
In your if statement where that alert is the answer, you're not checking equality of the variable, you're assigning it a value. You need to add one or two equals signs to that conditional check for it to be valid.
You have:
if (correctAnswers = 5) {
alert('You know me extremely well. You get a GOLD medal!');
} //rest of your if/else if here
You need:
if (correctAnswers == 5) {
alert('You know me extremely well. You get a GOLD medal!');
} //rest of your if/else if here
Or:
if (correctAnswers === 5) {
alert('You know me extremely well. You get a GOLD medal!');
} //rest of your if/else if here
If you want, though the === isn't 100% necessary (== will suffice, as you don't really need to check type as well).
Try that and see if it helps.
Erik
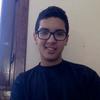
Mohammed Khalil Ait Brahim
9,539 PointsIn the condition you did
if (correctAnswers = 5) {
alert('You know me extremely well. You get a GOLD medal!');
}
Instead of Three equal signs :
if (correctAnswers === 5) {
alert('You know me extremely well. You get a GOLD medal!');
}
So what you were actually doing is assigning the value of 5 to correctAnswers and checking if 5 was a true value which is always the case
Zach Allan
19,452 PointsZach Allan
19,452 PointsAwesome! Thanks Erik.