Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial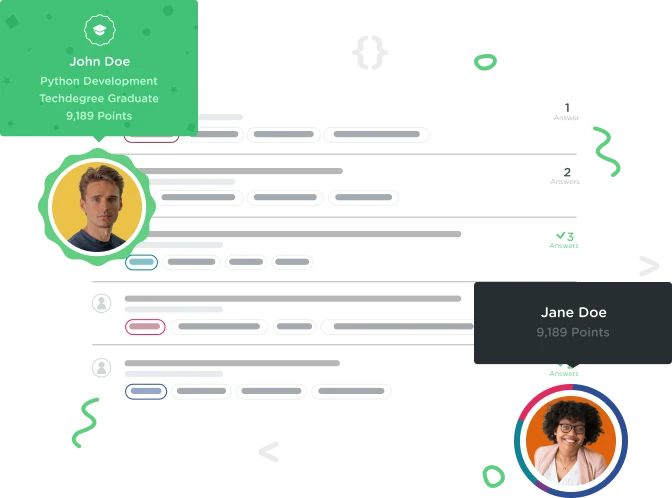

Nate Hawk
13,229 PointsWhat is wrong with my code? I feel like there is not enough info in this challenge to complete.
I keep getting this message.
You need to use bulk_create
to create the Review objects.; Make sure you create three Reviews.; Make sure you provide ratings of 2, 3, and 5.
from . import models
def fix_25():
product = Product.objects.filter(id=1)
Review.objects.bulk_create([
Review(product=product, rating=2),
Review(product=product, rating=3),
Review(product=product, rating=5)
])
3 Answers
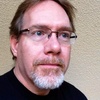
Chris Freeman
Treehouse Moderator 68,460 PointsSince models
was imported, Review
must be referenced using models.Review
. Also Product
should also be prefixed with "models.
"
Be sure to add the prefix everywhere:
from . import models
def fix_25():
product = models.Product.objects.get(id=1)
models.Review.objects.bulk_create([
models.Review(product=product, rating=2),
models.Review(product=product, rating=3),
models.Review(product=product, rating=5)
])
You could also change the import to:
from .models import Product, Review
The following also passes:
from .models import Product, Review
def fix_25():
product = Product.objects.get(id=1)
Review.objects.bulk_create([
Review(product=product, rating=2),
Review(product=product, rating=3),
Review(product=product, rating=5)
])

Nate Hawk
13,229 PointsIt won't let me import from models, so I used from models import Review
I am still getting the same error though. My code now looks like
from . import models
from products.models import Product, Review
def fix_25():
product = Product.objects.filter(id=1)
Review.objects.bulk_create([
Review(product=product, rating=2),
Review(product=product, rating=3),
Review(product=product, rating=5)
])
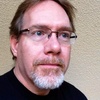
Chris Freeman
Treehouse Moderator 68,460 PointsThe better solution is to prefix Review and Product with "models.
"

Nate Hawk
13,229 PointsI have tried that as well. I can't really tell what's wrong, its not giving me enough info. What it says is "Bummer! You need to use bulk_create
to create the Review objects.; Make sure you create three Reviews.; Make sure you provide ratings of 2, 3, and 5."
use bulk create: check; Review objects: check; ratings of 2, 3 & 5: check.
from . import models
def fix_25():
product = models.Product.objects.filter(id=1)
models.Review.objects.bulk_create([
Review(product=product, rating=2),
Review(product=product, rating=3),
Review(product=product, rating=5)
])
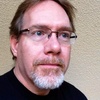
Chris Freeman
Treehouse Moderator 68,460 PointsBe sure to add prefix everywhere. See updated Annswer.

Nate Hawk
13,229 PointsThis is crazy, that is still not working. It feels like I just need all of the code from models, because right now I am just guessing.
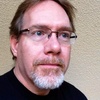
Chris Freeman
Treehouse Moderator 68,460 PointsSorry Nate, I missed a different error in the code. You need to use .get()
instead of .filter()
. Using .filter()
returns a list where .get()
returns the single item.
If .filter.()
was left in the code, then the references to product
(now a list) would need to be changed to product[0]
to get the first item of the list.
I've updated my Answer again.
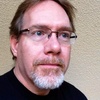
Chris Freeman
Treehouse Moderator 68,460 PointsSorry for the iterations. Working on my iPhone to run challenges is sub-optimal.
Nate Hawk
13,229 PointsNate Hawk
13,229 PointsYes, there we go. Thank you Chris, I knew I was missing something little yet fundamentally important in my code.