Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial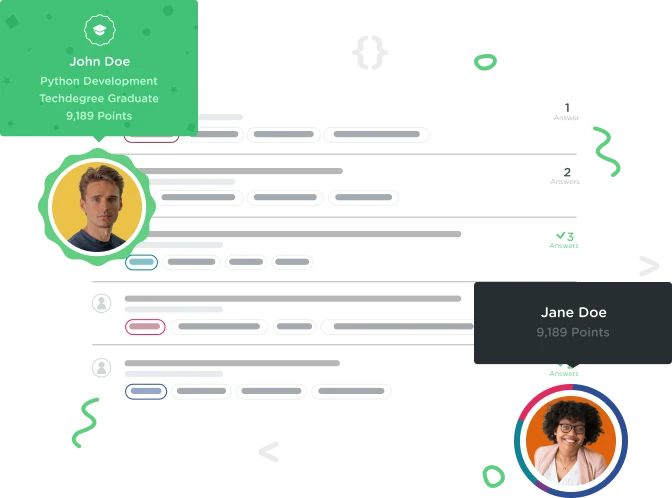

newpal
1,717 PointsWhat is wrong with my code please?
Let's use str to turn Python code into Morse code! OK, not really, but we can turn class instances into a representation of their Morse code counterparts.
I want you to add a str method to the Letter class that loops through the pattern attribute of an instance and returns "dot" for every "." (period) and "dash" for every "_" (underscore). Join them with a hyphen.
I've included an S class as an example (I'll generate the others when I test your code) and it's str output should be "dot-dot-dot".
class Letter:
outcome1 = ""
outcome2 = ""
def __init__(self, pattern=None):
self.pattern = pattern
def __str__(self):
for attribute in self.pattern:
if attribute == '.':
outcome1 += "dot-"
elif attribute == '_':
outcome2 += "dash-"
return outcome1 + outcome2
class S(Letter):
def __init__(self):
pattern = ['.', '.', '.']
super().__init__(pattern)
1 Answer
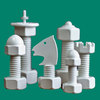
Steven Parker
231,269 PointsIt looks like this code will put all the dots together and all the dashes together, and then combine them so the dots come first. But for correct Morse, the order must be the same as the symbols in the pattern. For example, ".." should come out as "dash-dot-dot-dash", instead of "dot-dot-dash-dash-".
And it looks like you'll end up with a trailing hyphen after the last word, I'd expect the challenge to also consider that an error.

newpal
1,717 PointsThank you Steven for your explanation. I tried to solve the issues you pointed out. However, the code is till not passing. class Letter: outcome = "" def init(self, pattern=None): self.pattern = pattern def str(self): for attribute in self.pattern: if attribute == '.': outcome += "dot" if attribute != self.pattern[-1]: outcome += "-" elif attribute == '_': outcome += "dash" if attribute != self.pattern[-1]: outcome += "-" return outcome
class S(Letter): def init(self): pattern = ['.', '.', '.'] super().init(pattern)

newpal
1,717 Pointsclass Letter:
outcome = ""
def __init__(self, pattern=None):
self.pattern = pattern
def __str__(self, pattern):
for attribute in self.pattern:
if attribute == '.':
outcome += "dot"
if attribute != self.pattern[-1]:
outcome += "-"
elif attribute == '_':
outcome += "dash"
if attribute != self.pattern[-1]:
outcome += "-"
return outcome
class S(Letter):
def __init__(self):
pattern = ['.', '.', '.']
super().__init__(pattern)
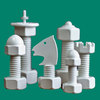
Steven Parker
231,269 PointsThis time, it looks like a hyphen will only be added it the current item is different from the last one. But that would mean a pattern like "S" would come out as "dotdotdot". The original idea of adding a hyphen every time wasn't bad, you'd just need to "slice" the last one off.
Also, the initialization of "outcome" seems to have gotten lost in the revision. And the function should not require an argument since the "pattern" is already in the instance.

newpal
1,717 PointsThanks Steven! What about this code:
class Letter:
outcome = ""
def __init__(self, pattern=None):
self.pattern = pattern
def __str__(self):
for attribute in self.pattern:
if attribute == '.':
outcome += "dot-"
elif attribute == '_':
outcome += "dash-"
outcome_list = list(outcome)
outcome_list.pop()
outcome = str(outcome_list)
return outcome
class S(Letter):
def __init__(self):
pattern = ['.', '.', '.']
super().__init__(pattern)
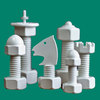
Steven Parker
231,269 PointsConverting a string into a list like that probably doesn't do what you're expecting. You might need to use "split" for that.
But you don't need to do all the conversion. That's why i was hinting that you might need to "slice" off the last character.

newpal
1,717 PointsHow do you slice off the last character? I tried slicing off the last character in the code below:
class Letter:
output = ""
def __init__(self, pattern=None):
self.pattern = pattern
def __str__(self):
for item in self.pattern:
if item == '.':
output += "dot-"
elif item == '_':
output += "dash-"
output = output[:-1]
return output
class S(Letter):
def __init__(self):
pattern = ['.', '.', '.']
super().__init__(pattern)

newpal
1,717 PointsMy code still throws an error!
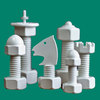
Steven Parker
231,269 PointsThe "slice" is exactly right. The only remaining issue is that the initialization of "output" needs to be moved into the function so it will start fresh each time the function is used.
Otherwise, I think you've got it!

newpal
1,717 PointsThank you Steven for your comments. It took me a while to solve this challenge but my code solves the challenge now! Thank you for your help!
newpal
1,717 Pointsnewpal
1,717 PointsThanks Steven! What about this code: