Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial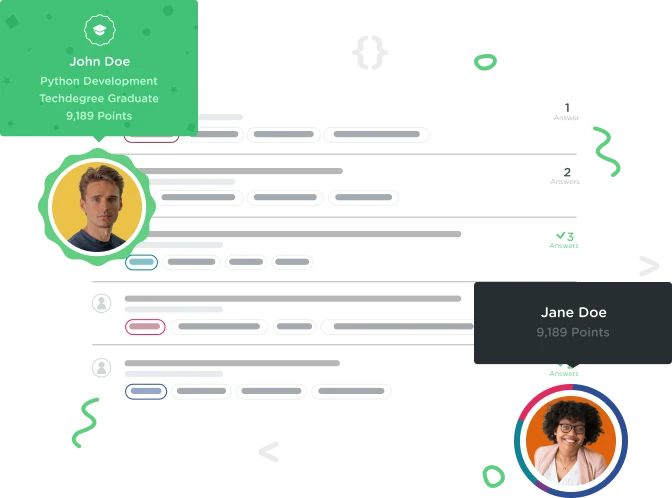

kori redman
4,275 PointsWhat is wrong with my code? Please help!
Here is what I currently have written.
def check_speed(car_speed) car_speed = get.chomp.to_i safe = (car_speed <= 40) && (car_speed >= 50) return safe end
def check_speed(car_speed)
car_speed = get.chomp.to_i
safe = (car_speed <= 40) && (car_speed >= 50)
return safe
end
3 Answers
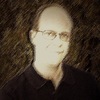
Jason Anders
Treehouse Moderator 145,860 PointsHi Kori and Welcome to Treehouse.
There are a few things wrong with your code.
(1) - I'm not sure why you are using a get.chomp.to_i
-- it is not asked for and is not needed. (2) - you have your comparison operators backwards -- you want to check to see if the speed is greater than / equal to 40 and less than / equal to 50, and for this, you need an if
statement. (3) - you are returning a variable called safe instead of a string (needs "").
The corrected code will look like this:
def check_speed(car_speed)
if (car_speed >= 40 && car_speed <= 50)
return "safe"
end
end
I hope this makes sense and helps. Keep Coding! :)
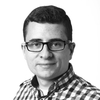
Tomasz Jaśkiewicz
5,399 PointsIf I may add something for the future. Look at this methods below:
def check_speed(car_speed)
case car_speed
when 40..50
return :safe
else
return :unsafe
end
end
It will return symbol :safe
if speed is in the range [40,50]
or will return :unsafe
otherwise.
What's my point here? There are a few actually:
- As you can see all possible cases are analysed here. If speed equals 40 it will return
:safe
if 20, 51, 60, 100 it will return:unsafe
. If you use method calledcheck_speed
you expect that it will inform you in all cases whether speed is safe or not. Considering previous method withif
statement it will returnnil
value if speed won't fit in the range.nil
is tricky and we shouldn't use it as return value. In this particular case you are checking speed and returning info about its safety. I usedcase when
here. You can simply add next ranges here (eg. define a new range51..60
and will return:too_high
. - I assume you'll be often using this function to check car speeds. You can use symbols instead of strings here. Each string has its own object id and if we have two the same symbols they have the same object id. Take a look here:
[1] pry(main)> "safe".object_id
=> 70324483105860
[2] pry(main)> "safe".object_id
=> 70324490479640
[3] pry(main)> :safe.object_id
=> 2875228
[4] pry(main)> :safe.object_id
=> 2875228
It means that you can simply save some memory :)
- If you want only to check if speed is safe you can write much simpler method:
def safe_speed?(car_speed)
40..50.include?(car_speed)
end
This method will return always boolean
value (true
or false
). Using ?
at the end of method name informs us that method will return only boolean values.
I'm using everywhere smth like this: 40..50
. If you're not familiar with it, it just creates a range from 40 (included) up to 50 (also included).
[1] pry(main)> (40..50).class
=> Range
[2] pry(main)> (40..50).count
=> 11
[3] pry(main)> (40..50).each{ |n| puts n }
40
41
42
43
44
45
46
47
48
49
50
=> 40..50
[4] pry(main)> (40..50).to_a
=> [40, 41, 42, 43, 44, 45, 46, 47, 48, 49, 50]
I hope, it will be useful in the future. Have fun with Ruby! :)

kori redman
4,275 PointsThis information is so thorough and I learned some more advanced code (..) to use in the future!
Thanks very much! :) I love coding!
kori redman
4,275 Pointskori redman
4,275 PointsThis is sooo helpful!!! Thanks so much!
I was using get.chomp for 2 reasons. 1. It is in the video just previous to this challenge (therefore thought it might secretly be needed and 2. I was trying to go above and beyond.
I be more mindful that Treehouse isn't trying to trick me, in the future! :)
Thanks again!
Jason Anders
Treehouse Moderator 145,860 PointsJason Anders
Treehouse Moderator 145,860 PointsYou are very welcome. :)
I hope you enjoy the rest of the Ruby track.
Ps. It is good practice to mark the answer that solved your question with "Best Answer", as this lets others in the community know that your post has been successfully resolved.