Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial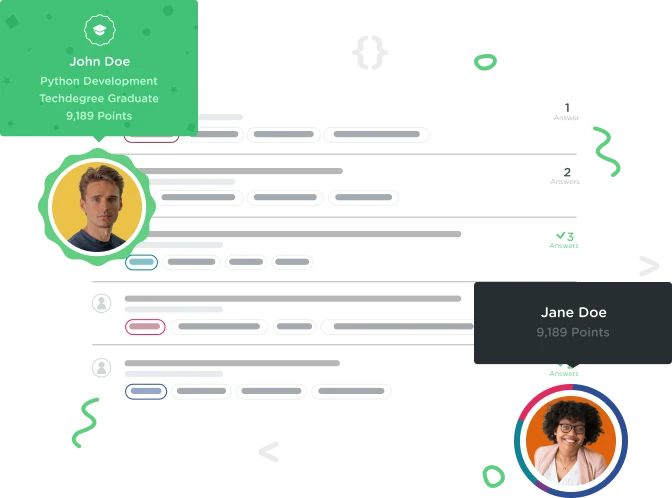
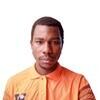
Joshua Ouma
20,865 PointsWhat is wrong with my code? Someone help
TypeError: Cannot read property 'source' of undefined at promises.js:35 at Array.map (<anonymous>) at generateHTML (promises.js:31) Below is my code:
const astrosUrl = 'http://api.open-notify.org/astros.json';
const wikiUrl = 'https://en.wikipedia.org/api/rest_v1/page/summary/';
const peopleList = document.getElementById('people');
const btn = document.querySelector('button');
function getJSON(url) {
return new Promise((resolve,reject)=>{
const xhr = new XMLHttpRequest();
xhr.open('GET', url);
xhr.onload = () => {
if(xhr.status === 200) {
let data = JSON.parse(xhr.responseText);
resolve(data);
}else{
reject(Error(xhr.statusText));
}
xhr.onerror = reject(Error("A network error occured"));
};
xhr.send();
});
}
function getProfiles(json) {
const profile = json.people.map( person => {
return getJSON(wikiUrl + person.name);
});
return Promise.all(profile);
}
function generateHTML(data) {
data.map( person =>{
const section = document.createElement('section');
peopleList.appendChild(section);
section.innerHTML = `
<img src=${person.thumbnail.source}>
<h2>${person.title}</h2>
<p>${person.description}</p>
<p>${person.extract}</p>
`;
});
}
btn.addEventListener('click', (event) => {
event.target.textContent = "Loading...";
getJSON(astrosUrl)
.then(getProfiles)
.then(generateHTML)
.catch(err => {
peopleList.innerHTML = "<h3>Something went wrong</h3>";
console.log(err)
})
.finally(event.target.remove());
});
2 Answers

Phil Wood
4,379 PointsHi Joshua,
The error is caused by 'thumbnail' not being defined. You are referencing a property that doesn't exist.
<img src=${person.thumbnail.source}>
The json you are sourcing from combining your two Urls is returning data - some of which do not contain 'thumbnail' You will have to put in a condition to test to see if the data is defined before trying to use it.
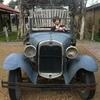
Brendan Moran
14,052 PointsTo troubleshoot this, use console.log(data)
to see what you are dealing with before the error occurs. Put it in the first line of the generateHTML
function. You might see that one of the profiles failed to come back from wikipedia. If that's the case, look at some of the other questions and answers on this video that deal with that problem.
Phil Wood
4,379 PointsPhil Wood
4,379 PointsYou could use something like this :