Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial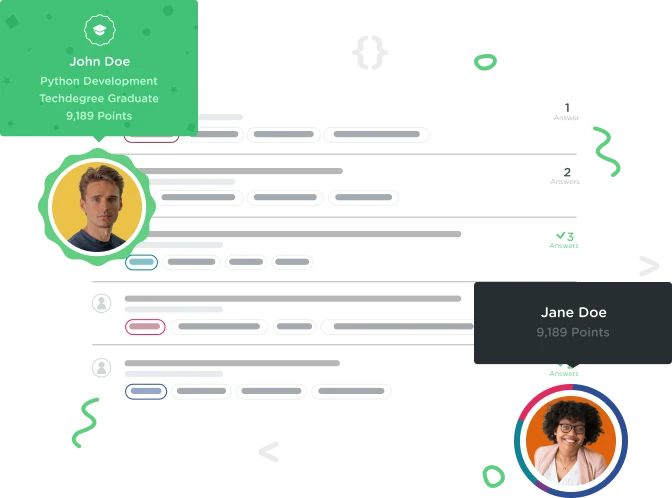
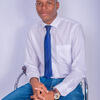
Tinotenda Mangarai
3,828 PointsWhat is wrong with my code. Someone help please. Been stuck for too long
Challenge Task 2 of 2 make an instance of your class named me. Then print() out the name attribute. Its not working
class Student:
name = "Tino"
me = Student()
print(me.name)
2 Answers

Fred Gablick
5,840 PointsYou're thinking of this as a function instead of an object class. You need to define the object outside of the class. This is quick and dirty, but I think you'll see it:
>>> class Student:
... pass
...
>>> me = Student()
>>> me.nickname = "Bonehead"
>>> print(me.nickname)
Bonehead
You can use an init function inside the class to define your attributes, but you still want to define the object outside of the class.
>>> class Student:
... def __init__(self, name):
... self.name = name
...
>>> me = Student("Bonehead")
>>> print(me.name)
Bonehead
>>>
I hope that helps.
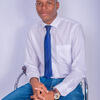
Tinotenda Mangarai
3,828 PointsThe problem was with indentation. Thank you all
Jeff Muday
Treehouse Moderator 28,720 PointsJeff Muday
Treehouse Moderator 28,720 PointsYou can fix your scenario with simple indentation changes. Python is very sensitive to indentation as it denotes the block level of code.