Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial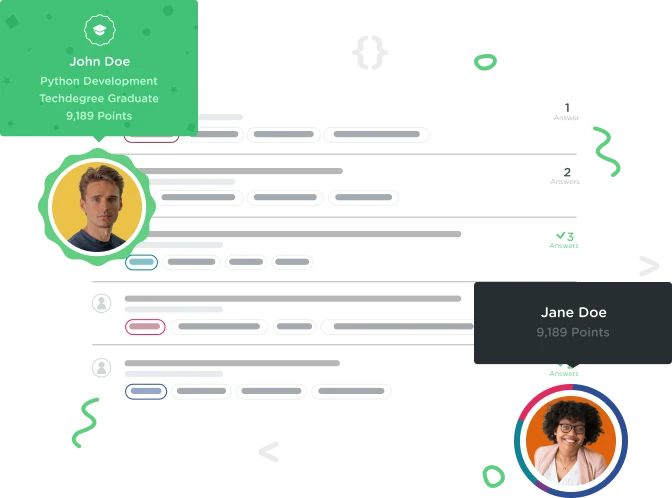
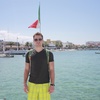
Michael Geatz
6,093 PointsWhat is wrong with my For Each loop?
I am having trouble with the getTileCount() method, and cannot complete the challenge. What is wrong with my For Each loop? Or is it something else? Thanks.
public class ScrabblePlayer {
private String mHand;
public ScrabblePlayer() {
mHand = "";
}
public String getHand() {
return mHand;
}
public void addTile(char tile) {
// Adds the tile to the hand of the player
mHand += tile;
}
public boolean hasTile(char tile) {
return mHand.indexOf(tile) > -1;
}
private int count;
public int getTileCount() {
//loop through the characters
for (char tile : mHand.toCharArray()) {
count = mHand.length();
//increment a counter if it matches
if (mHand.indexOf(tile) >= 0){
count++;
}
}
return count;
}
}
6 Answers
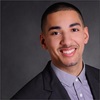
Mario Blokland
19,750 PointsHi Michael Geatz,
the first thing is that you didn't give your method a parameter, in this case the parameter "tile" (you can name it like you want to). Also, because we use the for loop, you don't need the .length method and even if you wanted to, you have made a small but big mistake :-).
The line with your command count = mHand.length(), is inside the for loop. So what happens is, that in every iteration, the count variable gets resetted to mHand.length. So the end result for the variable count would be:
- The value of mHand.length(), if nothing was found.
- The value of mHand.length(), if something was found, but nothing in the last iteration, because your variable gets resetted.
- The value 1, if something was found during the last iteration. (Doesn't matter if something was found before or not)
What I personally would do is:
- Put the count variable inside the method (since it is only needed by this method). But you could leave it like it is. If you chose to put the variable count inside the method, don't forget to delete the access modifier, since it's not needed and you would get an error.
- Delete the command: count = mHand.length()
- Add the needed parameter
You are most probably good to go after those changes. let me know if you could manage to pass this excercise and if you understood what went wrong. I will gladly help you more if needed.

Craig Dennis
Treehouse TeacherUse a different name for the variable inside the for loop:
for (char letter : mHand.toCharArray()) {
// if letter is equal to tile then increment
That hint do the trick?
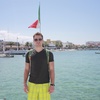
Michael Geatz
6,093 PointsSo I had to initialize count (setting it's value to 0 outside the for loop) within the method, and then I needed to use a different name for the variable inside the for loop (as Craig mentioned). Thanks everyone for the help!

ANDREA HEMPHILL
14,484 PointsI was stuck on this for a while as well. This hint does help. It would be nice if Treehouse had a hint section built into the code challenge. For example, if you hit submit 5 times then an option for a hint would pop up, and the hints get more detailed if necessary.
I was so close to solving this one!
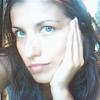
Alina Sirbeanu
992 PointsThis hint really work! Thank you Craig!

poltexious
Courses Plus Student 6,993 PointsJust to clear up things from Michael Geatz's work including the hint from Craig Dennis, this is how the method should look like:
public int getTileCount(char tile) {
int count = 0;
for (char letter : mHand.toCharArray()) {
if (letter == tile) {
count++;
}
}
return count;
}
Hope it cleared up things a little more.
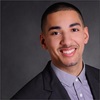
Mario Blokland
19,750 PointsHi,
I would try to avoid posting the answers directly as this is not as good for learning purposes as giving hints in my opinion. Others may just copy/paste the solution without even knowing what the problem was.

poltexious
Courses Plus Student 6,993 PointsWell, I was not suggesting that one should simply copy the solution and keep going. If one reads this forum thread I thought it was natural to end it with the solution - after all, it got quite messy IMO.
You make a good point, though! But in the end, it is up to others how they will handle this "problem" with someone clearing up things like I just did. Also, this is all about self discipline.
Everything in moderation, including moderation.
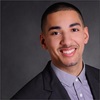
Mario Blokland
19,750 PointsI understand your point too, but like this, you really don't give a the viewer of this topic a choice. Let's say somebody only wants hints without the solution being presented directly and scrolls through this topic. There is no warning or spoiler etc. One just gets the solution and after that realizes that this is the solution. But that's just a different opinion and everybody has their own :-)

poltexious
Courses Plus Student 6,993 PointsYou are right, everyone learns in his own way, though.
It would be nice if the treehouse developers could make a new feature like 'spoiler alerts' in the forum or something like that. So when a user posts the solution, either the same user (or the user behind the thread) should mark the posted solution with a spoiler alert tag, so that each user reading that forum thread has to click the post with the spoiler alert tag in order to see it :-)
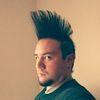
Ben Morse
6,068 PointsThanks poltexious. I couldn't understand why it wasn't working. I don't know if it's just me or that I'm just not quiet understanding the material and not trying to disrespect the instructor. If anything this is an area that I need to work on by other learning material. As students who is seeking to reach master level, we are always researching the area that we are studying, Java in this case. If I have not seen this post, I would have given up and never try again. I know I need to explore more on toCharArray() method usage.

ms2030
2,973 PointsBTW, just posting the answer helped me a LOT! My code was close but still came up with a compiler error that it wouldn't show me. I've seen this issue a lot. I must be making really strange mistakes. In many ways this is more difficult than programming in an IDE where it can help with simple syntax.
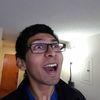
iftekhar uddin
4,194 Pointsmy code looks exactly like this (which I got on my own) but it says that the outcome was 1 when the outcome was expected to be 0.....i'm ripping my hair out trying to figure it out....

Dan Someone
1,320 PointsThanks very much for this discussion, I have been trying for 2 hours straight different things with this, and could not figure out what I was doing wrong!

Chetram Chinapana
1,793 PointsMario thanks for your your hints. As a beginner I fried my brain out last week on this one exercise. I finally gave up. I looked up the for loop and don't know why its not in the form of three arguments like for loops on the internet -- i googled it. Also, even with poltexious' answer I am trying to figure out why it works. The answer is there but the why it works is killing me but I am going to stare at it until I get the logic. Both of you are correct and that is why this forum work. Thanks to the both of you!
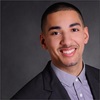
Mario Blokland
19,750 PointsThanks Chetram Chinapana,
When I began to learn programming I was in the same boat. I didn't know 'why' something worked and because of the terminology my brain was 'overflowed' quite quick :). But it is really like putting a puzzle together. If you know one piece, then eventually you will find another piece and things start to make sense. After a while those things will be much clearer. I promise.
The loop from above is just the enhanced version of the standard for loop. If you really wanted too, you could also implement this code as a standard for loop with 3 arguments but it probably won't get you passed this excercise because it expects the enhanced version.
The enhanced version is like saying this in the standard for loop:
for (int letter = 0; letter < mHand.toCharArray().length(); letter++) {
if (mHand.toCharArray()[letter] == tile) {
... do something
}
}
Can you get your head around this a little better now?

Chetram Chinapana
1,793 PointsMario, Thank you for that. It is a clearer now. Craig is doing a great job but he does not realize that his slow speed is fast speed to me but I appreciate his awesome effort in this MVP exercise, it is making my head hurt but that means I am learning. Thanks-again!

Rogelio Valdez
6,244 PointsMario Blokland: in the example that you just gave, is the "int letter = 0"...... the same as the "(mHands.toCharArray()[letter] == tile)?? Because I am still kind of confused but if it is the same, you are comparing an int (with the name letter) to a char (named tile). Or am I missing something??
Michael Geatz
6,093 PointsMichael Geatz
6,093 PointsHmm, it's still not giving me a pass. Here's what I have at this point:
I'm keeping the count variable outside the method for now, since I'm not sure if treehouse will have me use it in another method in the next challenge. I did try placing it inside the method (without the access modifier) for troubleshooting purposes, and it still doesn't give me a pass. Any more ideas? My brain is fried at this point, lol.
Mario Blokland
19,750 PointsMario Blokland
19,750 PointsHi Michael,
in this case you are only using the tile variable of the for loop because the name is the same as your parameter. This thing has to to with the scope of a variable. So in this case the first tile stays unsused. Craig's hint should do it.