Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial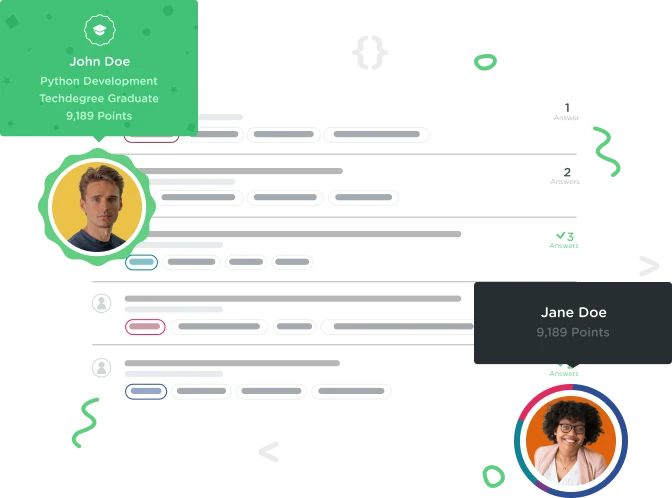

Tony Toscano
Courses Plus Student 13,109 PointsWhat is wrong with my Invader classes/interface?
There is an error that I'm getting when I compile this program. It states that that BasicInvader class doesn't have a definition for 'isNeutralized' , and no extension method 'isNeutralized' of type BasicInvader could be found. However, no method 'isNeutralized' is added to the BasicInvader class. My brain thinks its something a bit more simple than that to correct this error, but I'm at a loss of what this error happens to be.
ResurrectingInvader.cs
class ResurrectingInvader : IInvader { private BasicInvader _incarnation1; private StrongInvader _incarnation2;
public MapLocation Location => _incarnation1.isNeutralized ? _incarnation2.Location : _incarnation1.Location;
public bool HasScored => _incarnation1.HasScored || _incarnation2.HasScored;
public int Health => _incarnation1.isNeutralized ? _incarnation2.Health : _incarnation1.Health;
public bool IsNeutralized => _incarnation1.IsNeutralized && _incarnation2.IsNeutralized;
public bool IsActive => !(IsNeutralized || HasScored);
public ResurrectingInvader(Path path)
{
_incarnation1 = new BasicInvader(path);
_incarnation2 = new StrongInvader(path);
}
public void Move()
{
_incarnation1.Move();
_incarnation2.Move();
}
public void DecreaseHealth(int factor)
{
if(!_incarnation1.IsNeutralized)
{
_incarnation1.DecreaseHealth(factor);
}
else
{
_incarnation2.DecreaseHealth(factor);
}
}
Invader.cs
abstract class Invader : IInvader
{
private readonly Path _path;
private int _pathStep = 0;
protected virtual int StepSize { get; } = 1;
// Properties over accessor methods
public MapLocation Location => _path.GetLocationAt(_pathStep);
public abstract int Health { get; protected set; }
// True if the invader has reached the end of the path
public bool HasScored { get { return _pathStep >= _path.Length; } }
public bool IsNeutralized => Health <= 0;
public bool IsActive => !(IsNeutralized || HasScored);
// Constructor
public Invader(Path path)
{
_path = path;
}
public void Move() => _pathStep += StepSize;
public virtual void DecreaseHealth(int factor)
{
Health -= factor;
System.Console.WriteLine("Shot at and hit an invader!");
}
IInvader.cs namespace TreehouseDefense { interface IMappable { MapLocation Location { get; } }
interface IMovable { void Move(); }
interface IInvader : IMappable, IMovable
{
bool HasScored { get; }
int Health { get; }
bool IsNeutralized { get; }
bool IsActive { get; }
void DecreaseHealth(int factor);
} }
Game.cs
using System;
namespace TreehouseDefense { class Game { public static void Main() { Map map = new Map(8,5);
try
{
Path path = new Path(
new [] {
new MapLocation(0, 2, map),
new MapLocation(1, 2, map),
new MapLocation(2, 2, map),
new MapLocation(3, 2, map),
new MapLocation(4, 2, map),
new MapLocation(5, 2, map),
new MapLocation(6, 2, map),
new MapLocation(7, 2, map)
}
);
IInvader[] invaders =
{
new ShieldedInvader(path),
new FastInvader(path),
new StrongInvader(path),
new BasicInvader(path),
new ResurrectingInvader(path)
};
Tower[] towers = {
new Tower(new MapLocation(1,3,map)),
new StrongTower(new MapLocation(3,3,map)),
new LongRangeTower(new MapLocation(5,3,map)),
};
Level level = new Level(invaders)
{
Towers = towers
};
bool playerWon = level.Play();
Console.WriteLine("Player " + (playerWon ? "won" : "lost"));
}
catch(OutOfBoundsException ex)
{
Console.WriteLine(ex.Message);
}
catch(TreehouseDefenseException)
{
Console.WriteLine("Unhandled TreehouseDefense Exception");
}
catch(Exception ex)
{
Console.WriteLine("Unhandled Exception: " + ex);
}
}
}
}

Tony Toscano
Courses Plus Student 13,109 PointsMy apologies for the chunky markup.
Will this work? (And thank you for the video about the Workspace)
https://w.trhou.se/wuriiz4m04
2 Answers
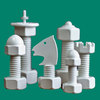
Steven Parker
230,274 PointsI'm wondering if you're compiling with the correct command.
I build the project from your snapshot with this command:
mcs -out:TreehouseDefense.exe *.cs
It built without any errors, so I ran it using this command:
mono TreehouseDefense.exe
After numerous hits and misses, all 5 invaders were neutralized and the program ended after declaring "Player won".
For future use, instructions for formatting your code can be found in the Markdown Cheatsheet below the "Add an Answer" area.

Tony Toscano
Courses Plus Student 13,109 PointsThank you Steven. Perhaps I was using incorrect syntax, or something. But it compiles with no issues now.

Mehmet Ali Ertörer
1,847 Pointsyou should (isNeutralized) to (IsNeutralized)
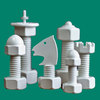
Steven Parker
230,274 PointsThat was already done in the workspace code. The workspace does not contain any instances of "isNeutralized" (with little "i").
Steven Parker
230,274 PointsSteven Parker
230,274 PointsAnalysis of a multi-file project like this would be easier if you make a snapshot of your workspace and share the link to it.