Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial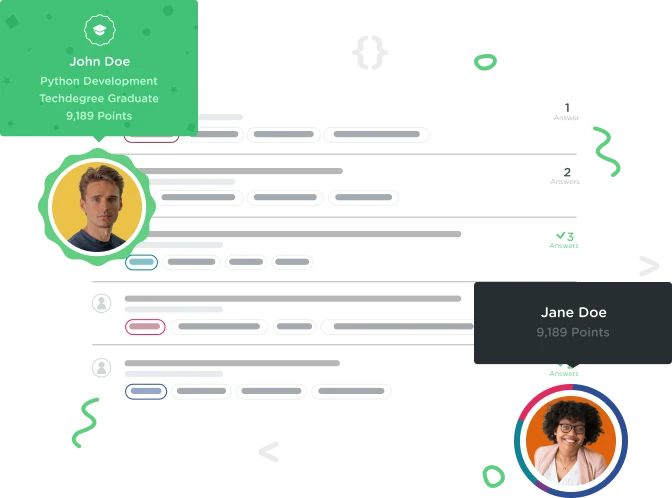

Adnan Nazir
Courses Plus Student 607 PointsWhat is wrong with my practice code?
This is my code
import android.content.Intent; import android.view.View;
public class MainActivity extends Activity {
private static final String KEY_USERENTRY = "KEY_USERENTRY";
public TextView mTextView;
@Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main);
mTextView = (TextView) findViewById(R.id.textView);
}
@Override
protected void onSaveInstanceState(Bundle outState) {
super.onSaveInstanceState(outState);
outState.putString(KEY_USERENTRY, mTextView);
}
@Override
protected void onRestoreInstanceState(Bundle savedInstanceState) {
super.onRestoreInstanceState(savedInstanceState);
mTextView = savedInstanceState.getString(KEY_USERENTRY);
}
}
that show this error
./MainActivity.java:21: error: cannot find symbol outState.putString(KEY_USERENTRY, mTextView); ^ symbol: method putString(String,TextView) location: variable outState of type Bundle ./MainActivity.java:29: error: cannot find symbol mTextView = savedInstanceState.getString(KEY_USERENTRY); ^ symbol: method getString(String) location: variable savedInstanceState of type Bundle 2 errors
1 Answer
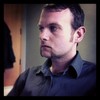
Conrad Spotts
11,768 PointsRight now, it looks like you're trying to save a TextView as a string, but it's not a String object, it's a TextView. I don't know what exercise this is for but here is my best guess as to why you're getting your error: you need get the text from your TextView object and convert it to a string to save it to the bundle. Then with onRestoreInstanceState , you need it converted back.
Whenever I come across problems like this in my code I try to google the error message (which didn't help me in your case.) The search that ended up being the winner was 'TextView Android onSaveInstanceState' and found a StackOverflow question that looks very much like what we're dealing with here. (here's the link http://stackoverflow.com/questions/15846005/saving-textview-with-onsaveinstancestate ) I hope this helps with your issue! Take a look at the example below where I've applied it to your code.
@Override
protected void onSaveInstanceState(Bundle outState) {
super.onSaveInstanceState(outState);
outState.putString(KEY_USERENTRY, mTextView.getText().toString();
}
@Override
protected void onRestoreInstanceState(Bundle savedInstanceState) {
super.onRestoreInstanceState(savedInstanceState);
mTextView.setText(savedInstanceState.getString(KEY_USERENTRY));
}