Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial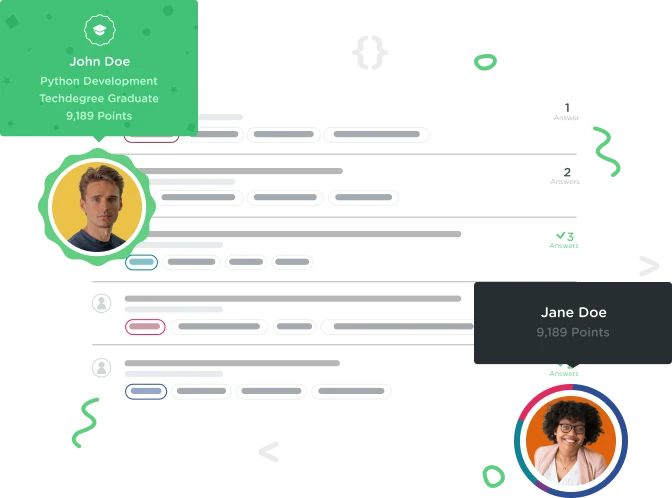
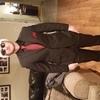
Daniel Hulin
1,852 PointsWhat is wrong with my setup when combining my hashes?
I tried to combine the calories to the grocery_list. Then that to the final hash. hat do I need to do?
grocery_item = { "item" => "Bread", "quantity" => 1, "brand" => "Treehouse Bread Company" }
calories = { "calories" => 100 }
grocery_item.merge(calories)
final_item = {}
final_item.merge!(grocery_item)
1 Answer
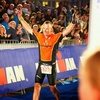
Steve Hunter
57,712 PointsHi Daniel,
You have written the correct line, you just didn't assign it to final_item
.
Try this;
final_item = grocery_item.merge(calories)
So you call the merge
method on one of the hashes and pass in the other hash. This then needs to be assigned to the new hash name.
You'd done that in your code!
Steve.
Daniel Hulin
1,852 PointsDaniel Hulin
1,852 PointsThanks! I understand what you did, but is there a way to merge like I was tring to do? EX: final_item.merge(grocery_item, calories)
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsNot with the
merge
method as that is expecting only one argument. This won't work:Also, I think you still need to assign the new value. So, the merge doesn't alter either hash, perhaps, but returns the altered/combined hash for you to handle how you choose.
So, here,
other_item
holds the same after themerge
; but the assignment stores the merged hash intonew_hash
.There may be alternative methods to use to add hashes together; but
merge
seems to want one parameter and its result will need assigning, if only to the original hash, for example. You could do this twice:Maybe this can be chained?
final_item = (final_item.merge(calories)).merge(grocery_item) # doesn't work
But that's not really adding much the more simple:
final_item = grocery_list.merge(calories)
Steve.