Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial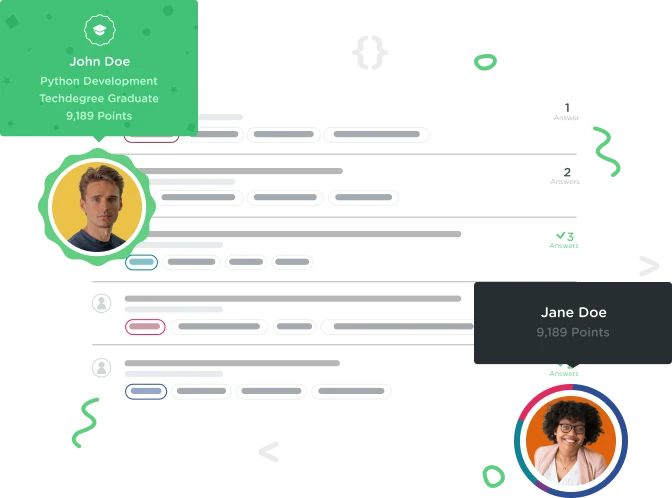

Varun Kukunoor
1,370 PointsWhat is wrong with my subclass?
The return line doesn't work and it claims that my self.title is not being initialized in the superclass. How do I fix this.
class Person {
let firstName: String
let lastName: String
init(firstName: String, lastName: String) {
self.firstName = firstName
self.lastName = lastName
}
func fullName() -> String {
return "\(firstName) \(lastName)"
}
}
// Enter your code below
class Doctor: Person {
var title: String
override init(firstName: String, lastName: String) {
super.init(firstName: firstName, lastName: lastName)
title = "Dr. "
}
override func fullName() -> String {
return "\(title) \(lastName)"
}
}
let someDoctor = Doctor.init(firstName: "Sam", lastName: "Smith").fullName()
1 Answer
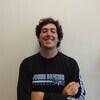
Charles Kenney
15,604 PointsSeeing as how the title property will always need to be equal to 'doctor' in case of this Doctor class, it is better practice to denote it's value in the class body rather than the initializer. This also avoids needlessly assigning the title as a variable in memory. Your class body should look like this:
class Doctor: Person {
let title = "Dr. "
Also, we need to assign the constant 'someDoctor' to an instance of Doctor. For this, we don't need to call the init method directly. Lastly, you need to delete the call to the fullName method at the end of the Doctor initialization, as it returns a string, and we want the full instance of the Doctor object. After these changes, your code should look like this:
class Doctor: Person {
let title = "Dr. "
override init(firstName: String, lastName: String) {
super.init(firstName: firstName, lastName: lastName)
}
override func fullName() -> String {
return "\(title) \(lastName)"
}
}
let someDoctor = Doctor(firstName: "Sam", lastName: "Smith")
Hope this helps. -Charles