Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial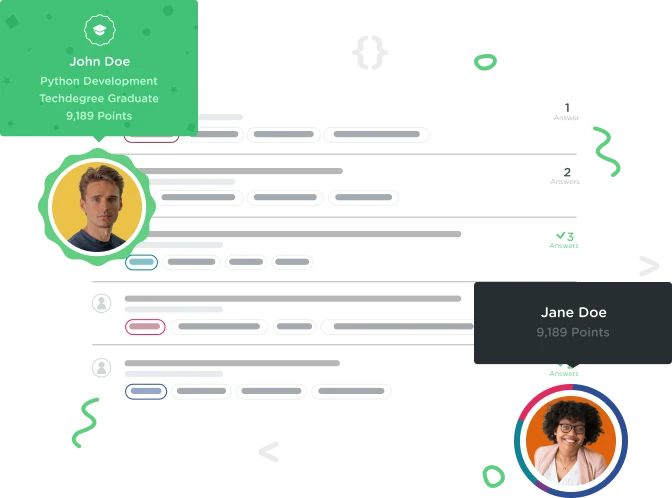

Alvaro Arroyo
1,084 PointsWhat is wrong with the code?
I can't figure out why it doesn't work. I've run it in IDLE and works perfectly.
def disemvowel(word):
vowels = ["a", "e", "i", "o", "u"]
index = 0
word = list(word)
for letter in word:
try:
word.remove(vowels[index])
word.remove(vowels[index].upper())
except ValueError:
pass
else:
index += 1
word = "".join(word)
return word
1 Answer
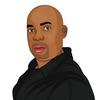
Quinton Gordon
10,985 PointsThere are a couple issues to address in this code. To start let's remove the "else" clause. In a Try...Except statement the "else" is used if you want to process code after the initial line but not catch any possible exceptions with the new code. In practice that else does not really add much and it's not being called the way you envisioned. So let us just junk it.
Then let's remove the statement checking for the uppercase as it will never run in practice unless there is a lowercase version of the vowel also present. So instead try lowercasing the word in advance before your loop.
You were trying to rely on the "else" to push the iteration along by increasing the index. Unfortunately that else would never be interpreted on a non-vowel and thus the index would never increase except for when a vowel was present on the iteration. So to push the iteration forward I added a step to the exception to increase the index and I dropped the "pass" as we really got no value from that.
def disemvowel(word):
vowels = ["a", "e", "i", "o", "u"]
index = 0
word = word.lower()
word = list(word)
for letter in word:
try:
word.remove(vowels[index])
except ValueError:
index += 1
word = "".join(word)
return word
Alvaro Arroyo
1,084 PointsAlvaro Arroyo
1,084 PointsThank you so much for taking the time to answer! That surely helps!!