Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial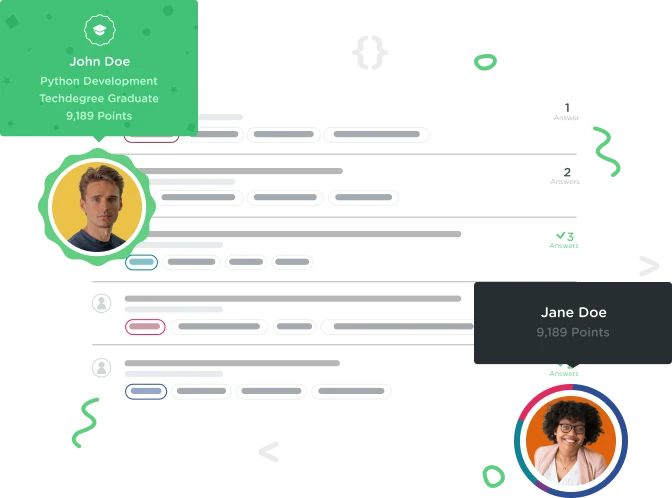
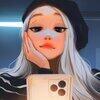
iclick
10,945 PointsWhat is wrong with the code?
./com/example/Blog.java:21: error: incompatible types: BlogPost cannot be converted to String for (String post : mPosts) { ^ Note: Some input files use unchecked or unsafe operations. Note: Recompile with -Xlint:unchecked for details. 1 error
6 Answers
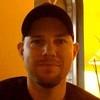
Jeremy Hill
29,567 PointsOkay, I had to find the challenge and jump into it to see what was going on. Don't feel so bad about having trouble on this one because I had a little bit of trouble trying to figure it out myself. You need to create two variables: a String to hold your category, and an Integer to hold your count. And same as before you need to loop through your blog posts so you want to use an enhanced for loop like this:
String category = "";
Integer count = 0;
for(BlogPost post : mPosts)
Next you want to set the String category to the post.getCategory() and then set the Integer to the value of the map for that category. Then go ahead and write in an if statement to check to see if the count is equal to null. Like this:
String category = "";
Integer count = 0;
for(BlogPost post: mPosts){
category = post.getCategory();
count = categoryCounts.get(category);
if(count == null){
count = 0;
}
}
Next, after your if statement you want to increment your count and then add the category and count to your map. Like this:
count++;
categoryCounts.put(category, count);
Last thing is to return your map but make sure that you do it after your for loop curly braces. Let me know if you need more info.
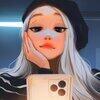
iclick
10,945 PointsAwesome Jeremy. I got it. Thanks a bunch :)
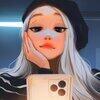
iclick
10,945 PointsGotcha Jeremy. Thank you. I got it working :)
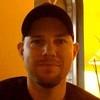
Jeremy Hill
29,567 PointsOkay good, you're welcome :)
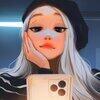
iclick
10,945 PointsJeremy can you help me with the error, please?
./com/example/Blog.java:32: error: for-each not applicable to expression type for (String category : bp.getCategory() ) { ^ required: array or java.lang.Iterable found: String Note: JavaTester.java uses unchecked or unsafe operations. Note: Recompile with -Xlint:unchecked for details. 1 error
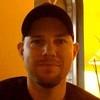
Jeremy Hill
29,567 PointsI'm away from my computer right now- I just have my phone and the battery is low, if you can paste all of your formatted code I can look at it in a little bit when I get back to my computer.
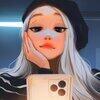
iclick
10,945 PointsThanks Jeremy
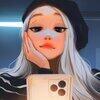
iclick
10,945 Pointspackage com.example;
import java.util.List; import java.util.Set; import java.util.TreeSet; import java.util.Map; import java.util.HashMap;
public class Blog { List<BlogPost> mPosts;
public Blog(List<BlogPost> posts) { mPosts = posts; }
public List<BlogPost> getPosts() { return mPosts; }
public Set<String> getAllAuthors() { Set<String> authors = new TreeSet<>(); for (BlogPost post: mPosts) { authors.add(post.getAuthor()); } return authors; }
public Map<String, Integer> getCategoryCounts(){ Map<String, Integer> categoryCounts = new HashMap<String, Integer>(); for (BlogPost bp : mPosts){ for (String category : bp.getCategory()){ Integer count = categoryCounts.get(category); if (count == null){ count = 0; } count++; categoryCounts.put(category, count); } } return categoryCounts; }
}
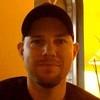
Jeremy Hill
29,567 PointsYou're welcome :)
Jeremy Hill
29,567 PointsJeremy Hill
29,567 PointsInstead of: String post : mPosts
Try:
for( BlogPost post : mPosts)
When you are trying to iterate through blogposts you need to store them in a variable of type BlogPost.