Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial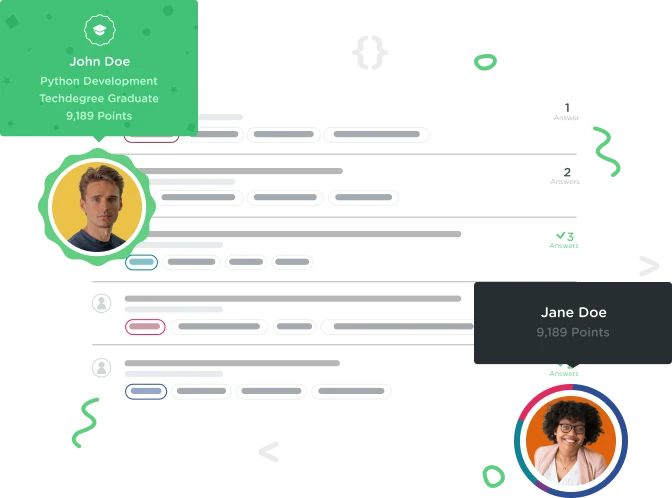

brandonlind2
7,823 PointsWhat is wrong with this answer?
I'm not sure exactly where I'm wrong
string input = Console.ReadLine();
int temp= int.Parse(input);
if ( temp < 21){
Console.Write("Too cold!");
}
else if(temp <= 22){
Console.Write("Just right!");
}
else {
Console.Write("Too hot!");
}
2 Answers
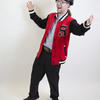
Sean Steberis
1,972 PointsIt looks like you're missing a semicolon on the end of this line,
Console.Write("Just right!")

Eduardo Parra San Jose
12,579 PointsHi,
I'll try to help you.
When the temperature is between 21 and 22, the string "Just right." should be written to the console, but you accidentally typed "Just right!" instead. So your code will work if you change it:
string input = Console.ReadLine();
int temp= int.Parse(input);
if ( temp < 21 ){
Console.Write("Too cold!");
}
else if( temp <= 22 ){
Console.Write("Just right.");
}
else if( temp > 22 ){
Console.Write("Too hot!");
}
Even though, the code now works, I think it will be clearer not to end an if statement with an else if clause. I'm sure there are better ways than the following one, but just to help and be as close as possible to what you write, I think you can refactor it as follows:
string input = Console.ReadLine();
int temp= int.Parse(input);
if ( temp < 21 ){
Console.Write("Too cold!");
}
else if ( temp <= 22 ){
Console.Write("Just right.");
}
else {
if( temp > 22 ) {
Console.Write("Too hot!");
}
}
I hope it helps. Best regards.
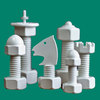
Steven Parker
229,783 PointsThe suggested additional "if" or "else if" is unnecessary.
The original "else" is just fine. The same clarity can be achieved without additional code with just a comment:
else /* temp > 22 */ {
Console.Write("Too hot!");
}