Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial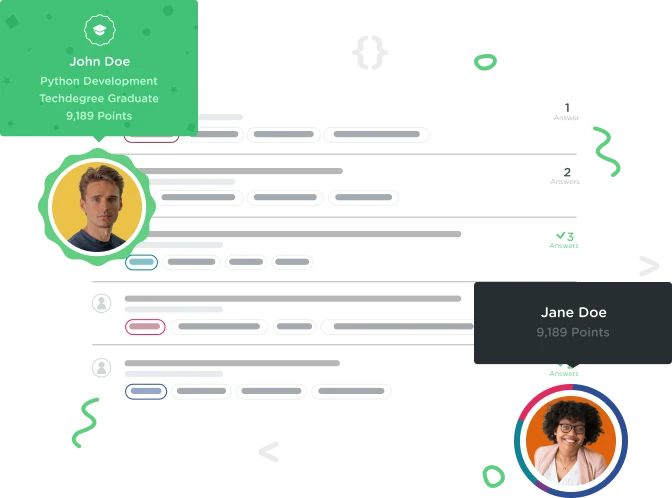

Chaya Feigelstock
2,429 PointsWhat is wrong with this code?
I don't remember it being mentioned that you can use a variable to end a slice but I'm not sure how else to do it?
def sillycase(string):
total = len(string)
total / 2 = index_counter
index_counter + 1 = end_of_lower
string[:end_of_lower] = lower_slice
lower_slice.lower() = lower_half
string[index_counter:] = upper_slice
upper_slice.upper() = upper_half
lower_half + upper_half = returned_value
return returned_value
def sillycase(string):
total = len(string)
total / 2 = index_counter
index_counter + 1 = end_of_lower
string[:end_of_lower] = lower_slice
lower_slice.lower() = lower_half
string[index_counter:] = upper_slice
upper_slice.upper() = upper_half
lower_half + upper_half = returned_value
return returned_value
1 Answer
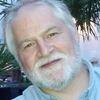
Jeff Muday
Treehouse Moderator 28,722 PointsI see you understand the concept! Just make sure the assignment statements are oriented from left to right. Once you have that solved, you are off to the races... You have a good methodology for solving code challenges, keep up the good work and the Pythonic power will be yours!
examine the code below, it's your code, just reoriented the assignment statements!
def sillycase(string):
total = len(string)
index_counter = int(total/2)
end_of_lower = index_counter
lower_slice = string[:end_of_lower]
lower_half = lower_slice.lower()
upper_slice = string[index_counter:]
upper_half = upper_slice.upper()
returned_value = lower_half + upper_half
return returned_value
Here is a simplified version of your code.
def sillycase(s):
midmark = int(len(s)/2)
return s[:midmark].lower() + s[midmark:].upper()