Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial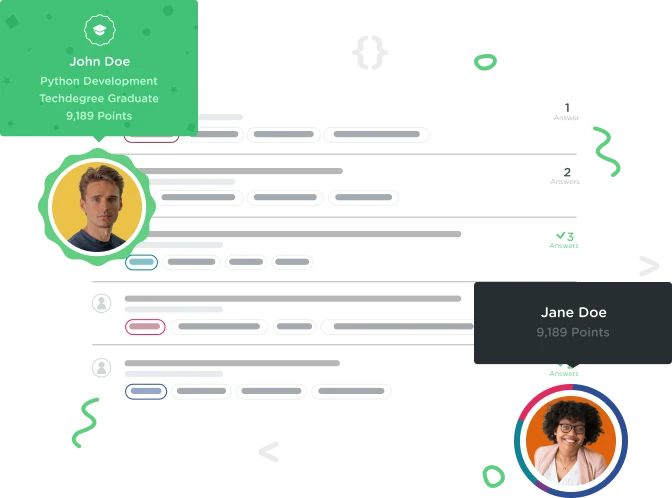

mateoniksic3
2,255 PointsWhat is wrong with this code?
What is wrong with my code?
def disemvowel(word):
char_list = list(word)
char_remove = set(('a','e','i','o','u'))
for char in char_list:
if char.lower() in char_remove:
char_list.remove(char)
word = ''.join(char_list)
return word
2 Answers
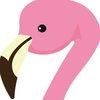
Dave StSomeWhere
19,870 PointsThe issue is that your are looping through a list and modifying that list while in the loop, so it is messing up the loop when you remove a character. It will skip the next character after a remove because you modified which character is at the next index entry value.
Probably the easiest fix is to just loop over word
(of course there are zillions of other options)...
Please let me know if you need additional explanation.

mateoniksic3
2,255 PointsYes I've got it now, it works.
Thanks a lot Dave.
mateoniksic3
2,255 Pointsmateoniksic3
2,255 PointsI'm coming from C programming language and this logic in python is killing me.
I tried this now and it still does not work...
def disemvowel(word): char_list = list(word) char_remove = ['a','e','i','o','u','A','E','I','O','U']
for i in (len(char_list)-1): if char_list[i] in char_remove: del char_list[i]
word = ''.join(char_list) return word
print(disemvowel('oARV'))
Could you post a solution maybe?
Dave StSomeWhere
19,870 PointsDave StSomeWhere
19,870 PointsSure thing...
Pretty soon you'll start to appreciate python - maybe???
You're over complicating things and your recent solution is still looping over
char_list
and messing up the index.Here's your original code with 1 small change to fix:
Hopefully, this makes sense
varlevi
8,113 Pointsvarlevi
8,113 PointsJust wondering: In your code, Dave, what does the keyword set do? Thanks in advance!
Dave StSomeWhere
19,870 PointsDave StSomeWhere
19,870 PointsHi Allison, That code is the OP's, not mine, not sure why they decided to use a
set()
. Also, I can't remember which course covered sets, but basically a set is similar to a list or dict and is a an un-ordered and unique collection. It also has some spiffy methods to do Venn diagram type functions like intersection - something like this set tutorial might provide some interesting info. When I did this challenge, I just used a list. Hope that answers your question.