Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial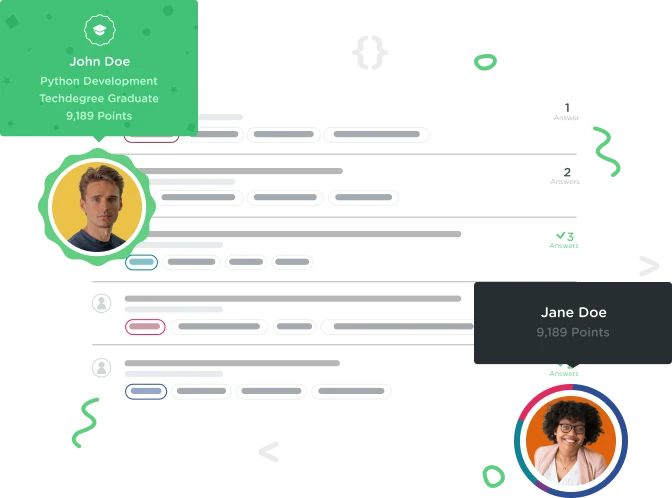

Rob Maslen
885 PointsWhat is wrong with this code?
When checking this code it says:
"Bummer: You must assert that the expected result matches the actual result."
What am i doing wrong?
Cheers!
<?php
use PHPUnit\Framework\TestCase;
class MathTest extends TestCase
{
/** @test */
public function Addition() {
$expected = 4;
$actual = 2 + 2;
assert('$actual === $expected');
}
}
?>
1 Answer

KRIS NIKOLAISEN
54,971 PointsI downloaded the project files and PigLatinTest.php gives an example of what the challenge is looking for:
<?php
use PHPUnit\Framework\TestCase;
//require_once __DIR__.'/../src/PigLating.php';
class PigLatinTest extends TestCase
{
/** @test */
function convertSingleStartingConsonantWordToPigLatin()
{
$word = 'test';
$expectedResult = 'esttay';
$pigLatin = new PigLatin();
$result = $pigLatin->convert($word);
$this->assertEquals(
$expectedResult,
$result
);
}
}
You should use assertEquals()
. There is an explanation and another example here. Or the documentation here.
Rob Maslen
885 PointsRob Maslen
885 PointsAh yeah that works thanks very much! :-)