Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial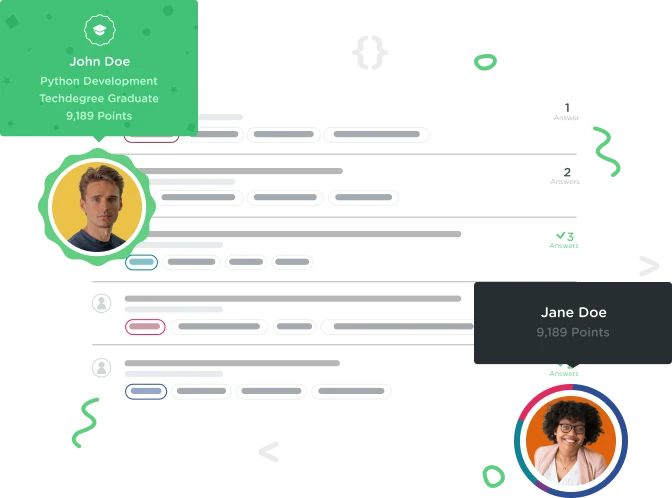

Forrest Pollard
1,988 PointsWhat is wrong with this code?
i thought i did a good job by trying to improve the number game by writing different code from the one shown in the notes of this video. here is my code:
let correctGuess = false;
const number = 6;
var guess = prompt("Guess a number between 1 & 10 to win a prize!");
if ( +guess === number ) {
correctGuess = true
} else if ( +guess > number ) {
alert(`Incorrect, The number is lower than ${guess}. Guess again`);
var newGuess = prompt("LAST CHANCE OR YOU FAAAAAAIL");
if ( +guess < number ) {
alert(`Incorrect, the number is greater than ${guess}. Guess again`);
var newGuess = prompt("LAST CHANCE OR YOU FAAAAAAIL");
}
}
if ( correctGuess ) {
alert("You win, GG2EZ")
} else if ( +newGuess === number ) {
alert("You win, GG2EZ");
if ( +newGuess != number ) {
alert(`You have performed a critical fail! The number was ${number}`);
}
}
however when I run it, it says there is an "Unexpected token: { at line 10" it is the function bracket for the end of the first if-else block. I am unsure why
EDIT; i have fixed the unexpected token problem by changing how the if-else was structured with the function brackets, but i dont fully understand why it works so would like an answer to that and will leave my question up, because even though it works im not sure why lol. Also, when i run this code, the only if-else thing that works currently is if you input a higher number than 6. This code challenge is kicking my butt
let correctGuess = false;
const number = 6;
let guess = +prompt("Guess a number between 1 & 10 to win a prize!");
let newGuess;
if ( guess === number ) {
correctGuess = true;
}
else {
if ( guess > number ) {
alert(`Incorrect, The number is lower than ${guess}. Guess again`);
}
if ( guess < number ) {
alert(`Incorrect, the number is greater than ${guess}. Guess again`);
}
newGuess = +prompt("LAST CHANCE OR YOU FAAAAAAIL");
}
if ( correctGuess ) {
alert("You win, GG2EZ");
}
else if ( newGuess === number ) {
alert("You win, GG2EZ");
}
if ( newGuess !== number ) {
alert(`You have performed a critical fail! The number was ${number}`);
}
2 Answers

Matthew Egginton
2,754 PointsYou are missing a few semicolons, and the end of the code you open a "else if " statement, but you have nested the final if statement inside it so the ending message wont ever execute.
Compare with fixed code here and see if you can get it to work :)
let correctGuess = false;
const number = 6;
var guess = prompt("Guess a number between 1 & 10 to win a prize!");
if (+guess === number) {
correctGuess = true;
}
else if (+guess > number) {
alert(`Incorrect, The number is lower than ${guess}. Guess again`);
var newGuess = prompt("LAST CHANCE OR YOU FAAAAAAIL");
}
if (+guess < number) {
alert(`Incorrect, the number is greater than ${guess}. Guess again`);
var newGuess = prompt("LAST CHANCE OR YOU FAAAAAAIL");
}
if (correctGuess) {
alert("You win, GG2EZ");
}
else if (+newGuess === number) {
alert("You win, GG2EZ");
}
if (+newGuess != number) {
alert(`You have performed a critical fail! The number was ${number}`);
}
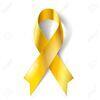
Mike Mike
Full Stack JavaScript Techdegree Student 10,055 PointsI fixed up up your code so it doesn't have that "you performed a critical fail" at the end, by fixing the if statement at the end
if ( newGuess !== number ) {
alert(`You have performed a critical fail! The number was ${number}`);
}
to this
let correctGuess = false;
const number = 6;
let guess = prompt("Guess a number between 1 & 10 to win a prize!");
let newGuess;
if ( +guess === number ) {
correctGuess = true;
}
else if ( +guess > number ) {
alert(`Incorrect, The number is lower than ${guess}. Guess again`);
newGuess = +prompt("LAST CHANCE OR YOU FAAAAAAIL");
}
else if ( +guess < number ) {
alert(`Incorrect, the number is greater than ${guess}. Guess again`);
newGuess = +prompt("LAST CHANCE OR YOU FAAAAAAIL");
}
if ( correctGuess ) {
alert("You win, GG2EZ");
}
else if ( newGuess === number ) {
alert("You win, GG2EZ");
} else {
alert(`You have performed a critical fail! The number was ${number}`);
}
There's no need to write another if statement because the else will automatically pick it if it's false. I hoped this helped.