Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial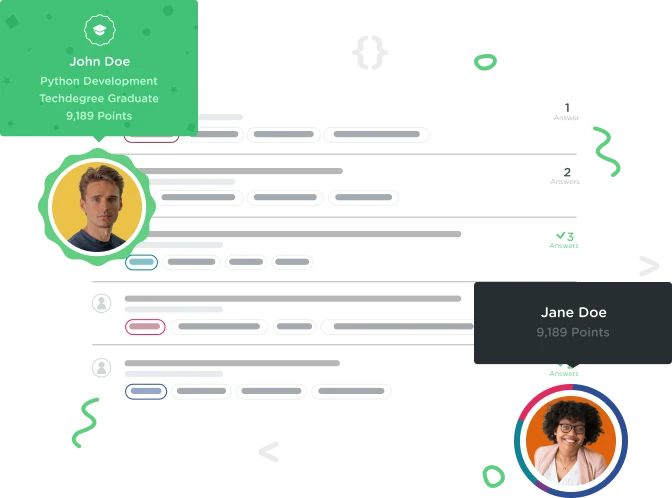

Frincu Tudor
993 PointsWhat is wrong with this code ?
I can't figure what is wrong with this code.... The quizz told me to add another EatFly class with 2 integer parameters and to return true if the frog length is longer then the fly distance and if the reaction time of the frog is faster then the fly reaction time. When i try to verify if i did it right it says : " Bummer! Did you create a new Eatfly method with the distanceToFly and flyReactionTime parameters"
namespace Treehouse.CodeChallenges
{
class Frog
{
public readonly int TongueLength;
public readonly int ReactionTime;
public Frog(int tongueLength , int reactionTime)
{
TongueLength = tongueLength;
ReactionTime= reactionTime;
}
public bool EatFly(int distanceToFly)
{
return TongueLength >= distanceToFly;
}
public bool EatFly(int distanceToFly , int flyReactionTime)
{
return Eatfly(distanceToFly) && (ReactionTime <= flyReactionTime);
}
}
}
4 Answers

Kristian Gausel
14,661 PointsYou didn't capitalize the methodcall to EatFly:
return Eatfly(distanceToFly) && (ReactionTime <= flyReactionTime);
Should be
return EatFly(distanceToFly) && (ReactionTime <= flyReactionTime);

Frincu Tudor
993 PointsThat worked. Thank you very much sir.

Kristian Gausel
14,661 PointsPlease mark as best answer if you are satisfied =) and no problem
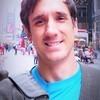
johnamann
14,064 PointsI ran into an issue with the conditions in the second method:
public bool EatFly(int distanceToFly, int flyReactionTime)
{
return TongueLength >= distanceToFly && ReactionTime >= flyReactionTime;
}
Strangely, the code was correct after changing the logic, requiring the frog's reaction time to be less than or equal to the fly's!
ReactionTIme <= flyReactionTime;

Nicholas Wallen
12,278 PointsThis is correct. The question and the answer do not match.

abdirahman yusuf
1,031 Points namespace Treehouse.CodeChallenges
{
class Frog
{
public readonly int TongueLength;
public readonly int ReactionTime;
public Frog(int tongueLength, int reactionTime)
{
TongueLength = tongueLength;
ReactionTime = reactionTime;
}
public bool EatFly(int distanceToFly)
{
return TongueLength >= distanceToFly;
}
public bool EatFly(int distanceToFly, int flyReactionTime) {
return TongueLength >= distanceToFly && ReactionTime <= flyReactionTime;
}
}
}
Frincu Tudor
993 PointsFrincu Tudor
993 PointsThank you for the answer , but i still have the same problem.....it keeps telling me " Bummer! Did you create a new Eatfly method with the distanceToFly and flyReactionTime parameters"
Kristian Gausel
14,661 PointsKristian Gausel
14,661 PointsTry that then, I removed a space here between the parameters:
public bool EatFly(int distanceToFly, int flyReactionTime)
Adam Leven
2,225 PointsAdam Leven
2,225 PointsThe second set of parenthesis aren't needed.