Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial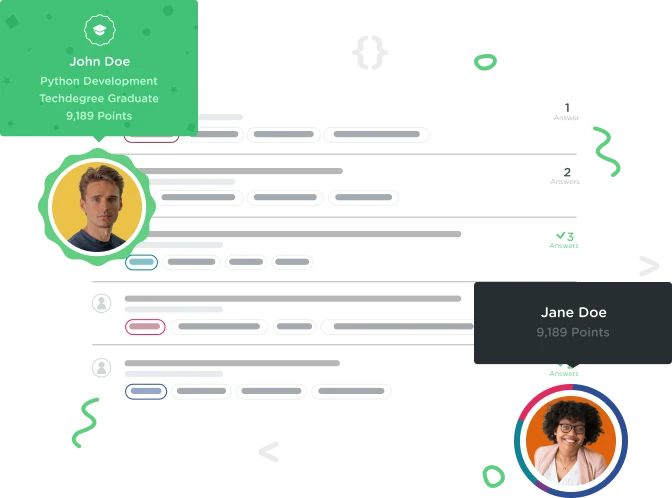

Jackson Monk
4,527 PointsWhat is wrong with this code?
I can't figure out why the numbers in this birthday calculator are so messed up. They aren't at all what they should be, so my math must be wrong. Also, when I print the value of years, it says it equals 0. I can't figure it out and I'm at the point where I need some help.
// beginning the program
var name = prompt("What is your name?"); console.log(name);
window.onload = function() { var month; var months = 0; var weeks = 0; var day; var days = 0; var year; var years = 0; var age = 0; var m; var d; var y;
function first() {
month = prompt(name + ", what month were you born?");
console.log(month);
month = parseInt(month);
if (month >= 1 && month <= 12) {
third();
}
else {
alert("That value is not representative of a month. Please restart the program and try again");
}
m = new Date();
months = m.getMonth();
months = parseInt(months);
}
weeks = days / 7;
function third() {
day = prompt(name + ", what day were you born?");
console.log(day);
day = parseInt(day);
if (month == 1) {
if (day >= 1 && day <= 31) {
fourth();
}
else {
alert("That is not a day of this month. Please restart the program and try again");
}
}
else if (month == 2) {
days = 31 + days;
if (day >= 1 && day<= 29) {
fourth();
}
else {
alert("That is not a day of this month. Please restart the program and try again");
}
}
else if (month == 3) {
days = 31 + 28.25 + days;
if (day >= 1 && day <= 31) {
fourth();
}
else {
alert("That is not a day of this month. Please restart the program and try again");
}
}
else if (month == 4) {
days = 31 + 28.25 + 31 + days;
if (day >= 1 && day <= 30) {
fourth();
}
else {
alert("That is not a day of this month. Please restart the program and try again");
}
}
else if (month == 5) {
days = 31 + 28.25 + 31 + 30 + days;
if (day >= 1 && day <= 31) {
fourth();
}
else {
alert("That is not a day of this month. Please restart the program and try again");
}
}
else if (month == 6) {
days = 31 + 28.25 + 31 + 30 + 31 + days;
if (day >= 1 && day <= 30) {
fourth();
}
else {
alert("That is not a day of this month. Please restart the program and try again");
}
}
else if (month == 7) {
days = 31 + 28.25 + 31 + 30 + 31 + 30 + days;
if (day >= 1 && day <= 31) {
fourth();
}
else {
alert("That is not a day of this month. Please restart the program and try again");
}
}
else if (month == 8) {
days = 31 + 28.25 + 31 + 30 + 31 + 30 + 31 + days;
if (day >= 1 && day <= 31) {
fourth();
}
else {
alert("That is not a day of this month. Please restart the program and try again");
}
}
else if (month == 9) {
days = 31 + 28.25 + 31 + 30 + 31 + 30 + 31 + 31 + days;
if (day >= 1 && day <= 30) {
fourth();
}
else {
alert("That is not a day of this month. Please restart the program and try again");
}
}
else if (month == 10) {
days = 31 + 28.25 + 31 + 30 + 31 + 30 + 31 + 31 + 30 + days;
if (day >= 1 && day <= 31) {
fourth();
}
else {
alert("That is not a day of this month. Please restart the program and try again");
}
}
else if (month == 11) {
days = 31 + 28.25 + 31 + 30 + 31 + 30 + 31 + 31 + 30 + 31 + days;
if (day >= 1 && day <= 30) {
fourth();
}
else {
alert("That is not a day of this month. Please restart the program and try again");
}
}
else if (month == 12) {
days = 31 + 28.25 + 31 + 30 + 31 + 30 + 31 + 31 + 30 + 31 + 30 + days;
if (day >= 1 && day <= 31) {
fourth();
}
else {
alert("That is not a day of this month. Please restart the program and try again");
}
}
d = new Date();
days = d.getDate();
days = parseInt(days);
}
function fourth() {
year = prompt(name + ", what year were you born?");
console.log(year);
year = parseInt(year);
if (year >= 0) {
final();
}
else {
alert("Invalid year. Please restart the program and enter a valid year");
}
age = years - year;
if (month > months) {
age = age - 1;
}
y = new Date();
years = y.getFullYear();
years = parseInt(years);
}
function final() {
document.write(name + ", you are " + (years - ((year - 1) * 365.25) + days ) + " days old");
document.write(", you are " + ((years - (year - 1) * 12) + month) + " months old");
document.write(", you are " + ((years - (year - 1) * 52) + weeks) + " weeks old");
document.write(", you are " + age + " years old");
}
first();
};
9 Answers
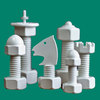
Steven Parker
231,269 PointsIt looks like you may have some sequence issues. In particular, each stage ("first", "third", etc) seems to call the next one right after validating the input. But the calculations needed for the output are not performed for each stage are not performed until the next stage function finishes and returns. So you probably want to call the other functions at the end of each stage.
Also, at least one calculation (weeks) is done between functions, which means it will occur before the prompts are used to get any input..
Finally, you can make posted code easier to read if you use the instructions for code formatting in the Markdown Cheatsheet pop-up below the "Add an Answer" area.
Or watch this video on code formatting.

Jackson Monk
4,527 PointsIf I call the functions at the end of each stage, they will run regardless of whether the user input a valid day, month, year. I only want the program to continue if the user input correct information.
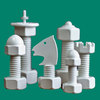
Steven Parker
231,269 PointsDepending on what behavior you want, you could possibly loop until you get valid input, and then do the calculations before going to the next stage.

Jackson Monk
4,527 PointsTrue, but I want it to end the program if the information is wrong. Also doing the calculations in a function before the next one would have you input a month, then output how many months old you are, before asking how many days old you are
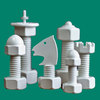
Steven Parker
231,269 PointsAs currently structured, doing the calculations would not cause any output. The output only occurs when the "final" function is called. And instead of looping on bad input, you can simply return instead to have the program end.

Jackson Monk
4,527 PointsYes I agree. But the final function is called after all the prompts have run and gotten valid answers
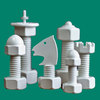
Steven Parker
231,269 PointsAfter the answers, yes — but before the calculations are made for the output.

Jackson Monk
4,527 PointsYou were right about the calculations. Once I stored them in separate variables and put those variables in the document.write command, I stopped getting negative numbers and just got 0. Now all I need to know is why months and years are equal to 0.
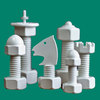
Steven Parker
231,269 PointsHave you changed the order around so the calculations are performed before the output is done? That was what gave the 0 before. You may need to post the current version of the code again if you still need help. Remember to use markdown for proper formatting.

Jackson Monk
4,527 PointsYes I did the calculations before the output is performed. Here is the updated code btw...
// beginning the program
var name = prompt("What is your name?"); console.log(name);
window.onload = function() { var month; var months = 0; var weeks = 0; var day; var days = 0; var year; var years = 0; var age = 0; var m; var d; var y; var resultm = 0; var resultd = 0; var resultw = 0;
function first() {
month = prompt(name + ", what month were you born?");
console.log(month);
month = parseInt(month);
if (month >= 1 && month <= 12) {
third();
}
else {
alert("That value is not representative of a month. Please restart the program and try again");
}
m = new Date();
months = m.getMonth();
months = parseInt(months);
resultm = ((years - (year - 1) * 12) + month);
}
function second() {
weeks = days / 7;
resultw = ((years - (year - 1) * 52) + weeks);
}
function third() {
day = prompt(name + ", what day were you born?");
console.log(day);
day = parseInt(day);
if (month == 1) {
if (day >= 1 && day <= 31) {
fourth();
}
else {
alert("That is not a day of this month. Please restart the program and try again");
}
}
else if (month == 2) {
days = 31 + days;
if (day >= 1 && day<= 29) {
fourth();
}
else {
alert("That is not a day of this month. Please restart the program and try again");
}
}
else if (month == 3) {
days = 31 + 28.25 + days;
if (day >= 1 && day <= 31) {
fourth();
}
else {
alert("That is not a day of this month. Please restart the program and try again");
}
}
else if (month == 4) {
days = 31 + 28.25 + 31 + days;
if (day >= 1 && day <= 30) {
fourth();
}
else {
alert("That is not a day of this month. Please restart the program and try again");
}
}
else if (month == 5) {
days = 31 + 28.25 + 31 + 30 + days;
if (day >= 1 && day <= 31) {
fourth();
}
else {
alert("That is not a day of this month. Please restart the program and try again");
}
}
else if (month == 6) {
days = 31 + 28.25 + 31 + 30 + 31 + days;
if (day >= 1 && day <= 30) {
fourth();
}
else {
alert("That is not a day of this month. Please restart the program and try again");
}
}
else if (month == 7) {
days = 31 + 28.25 + 31 + 30 + 31 + 30 + days;
if (day >= 1 && day <= 31) {
fourth();
}
else {
alert("That is not a day of this month. Please restart the program and try again");
}
}
else if (month == 8) {
days = 31 + 28.25 + 31 + 30 + 31 + 30 + 31 + days;
if (day >= 1 && day <= 31) {
fourth();
}
else {
alert("That is not a day of this month. Please restart the program and try again");
}
}
else if (month == 9) {
days = 31 + 28.25 + 31 + 30 + 31 + 30 + 31 + 31 + days;
if (day >= 1 && day <= 30) {
fourth();
}
else {
alert("That is not a day of this month. Please restart the program and try again");
}
}
else if (month == 10) {
days = 31 + 28.25 + 31 + 30 + 31 + 30 + 31 + 31 + 30 + days;
if (day >= 1 && day <= 31) {
fourth();
}
else {
alert("That is not a day of this month. Please restart the program and try again");
}
}
else if (month == 11) {
days = 31 + 28.25 + 31 + 30 + 31 + 30 + 31 + 31 + 30 + 31 + days;
if (day >= 1 && day <= 30) {
fourth();
}
else {
alert("That is not a day of this month. Please restart the program and try again");
}
}
else if (month == 12) {
days = 31 + 28.25 + 31 + 30 + 31 + 30 + 31 + 31 + 30 + 31 + 30 + days;
if (day >= 1 && day <= 31) {
fourth();
}
else {
alert("That is not a day of this month. Please restart the program and try again");
}
}
d = new Date();
days = d.getDate();
days = parseInt(days);
resultd = (years - ((year - 1) * 365.25) + days );
}
function fourth() {
year = prompt(name + ", what year were you born?");
console.log(year);
year = parseInt(year);
if (year >= 0) {
final();
}
else {
alert("Invalid year. Please restart the program and enter a valid year");
}
age = years - year;
if (month > (months + 1)) {
age = age - 1;
}
y = new Date();
years = y.getFullYear();
years = parseInt(years);
}
function final() {
document.write(name + ", you are " + age + " years old");
document.write(", you are " + resultm + " months old");
document.write(", you are " + resultw + " weeks old");
document.write(", you are " + resultd + " days old");
}
first();
};
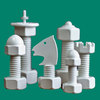
Steven Parker
231,269 PointsWhen posting code, use the instructions for code formatting in the Markdown Cheatsheet pop-up below the "Add an Answer" area.
Or watch this video on code formatting.
But the sequencing still looks essentially the same as the first time. The functions are still being called after validating the input but before performing the calculations on it. So when "final" gets called, everything is still 0.
Here's a pseudo-code version of one of the stages as they are now:
function xxx() {
prompt for input
if input validates
call the next stage
else
give error message
calculate the results
}
But what I was suggesting would be more like this:
function xxx() {
prompt for input
if input DOES NOT validate
give error message and return
calculate the results
THEN call the next stage
}

Jackson Monk
4,527 PointsOh so you're saying do the calculations and get date methods before the conditional statements?
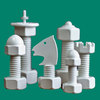
Steven Parker
231,269 PointsNo, but do them before calling the function for the next step.
Also, the "best answer" designation is usually given to the answer that contained the information most helpful to resolving your issue. Marking another question as "best answer" might be confusing to other readers.

Jackson Monk
4,527 PointsThere's just one more problem, but it's an easy fix. There appears to be an unmatched ( somewhere in the code. It says it's on line 196, but that line doesn't exist. I think I just need an extra eye to help point it out for me. Here's the new code...
// beginning the program
var name = prompt("What is your name?"); console.log(name);
window.onload = function() { var month; var months = 0; var weeks = 0; var day; var days = 0; var year; var years = 0; var age = 0; var m; var d; var y; var resultm = 0; var resultd = 0; var resultw = 0;
function first() {
month = prompt(name + ", what month were you born?");
console.log(month);
month = parseInt(month);
m = new Date();
months = m.getMonth();
months = parseInt(months);
resultm = ((years - (year - 1) * 12) + month);
if (month >= 1 && month <= 12) {
second();
}
else {
alert("That value is not representative of a month. Please restart the program and try again");
}
}
function second() {
weeks = days / 7;
resultw = ((years - (year - 1) * 52) + weeks);
third();
}
function third() {
day = prompt(name + ", what day were you born?");
console.log(day);
day = parseInt(day);
d = new Date();
days = d.getDate();
days = parseInt(days);
resultd = (years - ((year - 1) * 365.25) + days );
if (month == 1) {
if (day >= 1 && day <= 31) {
fourth();
}
else {
alert("That is not a day of this month. Please restart the program and try again");
}
}
else if (month == 2) {
days = 31 + days;
if (day >= 1 && day<= 29) {
fourth();
}
else {
alert("That is not a day of this month. Please restart the program and try again");
}
}
else if (month == 3) {
days = 31 + 28.25 + days;
if (day >= 1 && day <= 31) {
fourth();
}
else {
alert("That is not a day of this month. Please restart the program and try again");
}
}
else if (month == 4) {
days = 31 + 28.25 + 31 + days;
if (day >= 1 && day <= 30) {
fourth();
}
else {
alert("That is not a day of this month. Please restart the program and try again");
}
}
else if (month == 5) {
days = 31 + 28.25 + 31 + 30 + days;
if (day >= 1 && day <= 31) {
fourth();
}
else {
alert("That is not a day of this month. Please restart the program and try again");
}
}
else if (month == 6) {
days = 31 + 28.25 + 31 + 30 + 31 + days;
if (day >= 1 && day <= 30) {
fourth();
}
else {
alert("That is not a day of this month. Please restart the program and try again");
}
}
else if (month == 7) {
days = 31 + 28.25 + 31 + 30 + 31 + 30 + days;
if (day >= 1 && day <= 31) {
fourth();
}
else {
alert("That is not a day of this month. Please restart the program and try again");
}
}
else if (month == 8) {
days = 31 + 28.25 + 31 + 30 + 31 + 30 + 31 + days;
if (day >= 1 && day <= 31) {
fourth();
}
else {
alert("That is not a day of this month. Please restart the program and try again");
}
}
else if (month == 9) {
days = 31 + 28.25 + 31 + 30 + 31 + 30 + 31 + 31 + days;
if (day >= 1 && day <= 30) {
fourth();
}
else {
alert("That is not a day of this month. Please restart the program and try again");
}
}
else if (month == 10) {
days = 31 + 28.25 + 31 + 30 + 31 + 30 + 31 + 31 + 30 + days;
if (day >= 1 && day <= 31) {
fourth();
}
else {
alert("That is not a day of this month. Please restart the program and try again");
}
}
else if (month == 11) {
days = 31 + 28.25 + 31 + 30 + 31 + 30 + 31 + 31 + 30 + 31 + days;
if (day >= 1 && day <= 30) {
fourth();
}
else {
alert("That is not a day of this month. Please restart the program and try again");
}
}
else if (month == 12) {
days = 31 + 28.25 + 31 + 30 + 31 + 30 + 31 + 31 + 30 + 31 + 30 + days;
if (day >= 1 && day <= 31) {
fourth();
}
else {
alert("That is not a day of this month. Please restart the program and try again");
}
}
}
function fourth() {
year = prompt(name + ", what year were you born?");
console.log(year);
year = parseInt(year);
y = new Date();
years = y.getFullYear();
years = parseInt(years);
age = years - year;
if (month > (months + 1)) {
age = age - 1;
}
if (year >= 0) {
final();
}
else {
alert("Invalid year. Please restart the program and enter a valid year");
}
function final() {
document.write(name + ", you are " + age + " years old");
document.write(", you are " + resultm + " months old");
document.write(", you are " + resultw + " weeks old");
document.write(", you are " + resultd + " days old");
}
first();
};

Jackson Monk
4,527 PointsOkay I figured that out, and the years work, but the months, weeks, and days all return NaN