Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial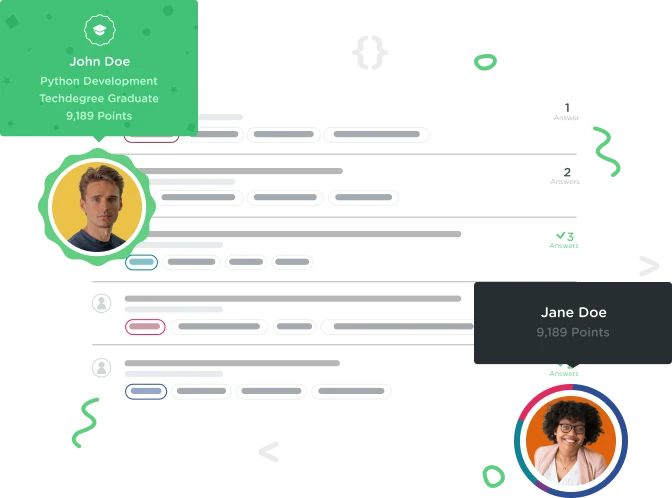

selcuk baran
3,526 PointsWhat may be the problem with the code below?
def members(dict,list): count=0 for item in list: if dict['item']==True: count=count+1 else: count=count return count
# You can check for dictionary membership using the
# "key in dict" syntax from lists.
### Example
# my_dict = {'apples': 1, 'bananas': 2, 'coconuts': 3}
# my_list = ['apples', 'coconuts', 'grapes', 'strawberries']
# members(my_dict, my_list) => 2
def members(dict,list):
count=0
for item in list:
if dict['item']==True:
count=count+1
else:
count=count
return count
1 Answer

Nate Sturgeon
2,255 PointsSo in addition to my comment above, there are a few other problems with your code:
First, your return count line is improperly indented such that it is outside your function. This will cause your code to fail.
Secondly, there is are some problems with how you've tried to test membership in your dictionary. First, item is a variable that's being reassigned on each for loop, so you shouldn't be putting it in quotes. As written, your code is just testing if the string "item" is a key in your dictionary over and over again, which is not what you want. However, removing the quotes reveals another problem...
When item is a key in your dictionary, everything works fine and count gets incremented by 1. However, when item is not a key in your dictionary, instead of being False and sending your if statement to the else clause, python throws an exception KeyError!
To fix this, you might try getting rid of your if ... else statement all-together and instead using a second for loop inside your first one. Try playing around with how to make that work.
Nate Sturgeon
2,255 PointsNate Sturgeon
2,255 Points*Very important!!! dict and list are built-in functions (aka keywords) in python! Do not use them as variables, as this will break their built-in functionality and most likely cause errors in your code! It's also very confusing to others. Change all your variables named dict and list to a_dict and a_list (or anything else other than python's reserved keywords). I believe there are other problems with your code, but go ahead and fix this right away and try to always remember it. It will save you many headaches down the road!