Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial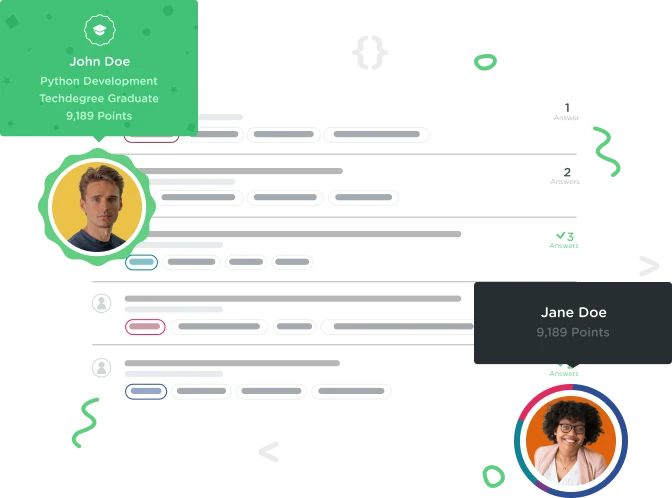
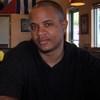
Kevin Ruddock
18,323 PointsWhat method or operation is not implemented?
The objective: Stub out the Calculator class so that the code compiles, but the test cases in CalculatorTests fail as expected.
The error: The method or operation is not implemented.
using System;
public class Calculator
{
public Calculator(double val)
{
throw new NotImplementedException();
}
public double Result{get;set;}
public void Add(double val)
{
throw new NotImplementedException();
}
}
using Xunit;
public class CalculatorTests
{
[Fact]
public void Initialization()
{
var expected = 1.1;
var target = new Calculator(1.1);
Assert.Equal(expected, target.Result, 1);
}
[Fact]
public void BasicAdd()
{
var target = new Calculator(1.1);
target.Add(2.2);
var expected = 3.3;
Assert.Equal(expected, target.Result, 1);
}
}
3 Answers
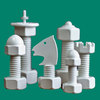
Steven Parker
231,236 PointsHere, you want them to be "implemented".
For the purposes of testing, you want the functions implemented but empty. Don't throw the exceptions, that's essentially the same as not having the function at all.
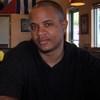
Kevin Ruddock
18,323 PointsMuch appreciated Steven!
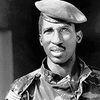
Henry Ojukwu
2,475 PointsHope this helps To Stub out a class for the purpose of running a test, you are basically required to write the constructor(s) and method(s) of the class, however, the method(s) should not be implemented as this is merely the red stage of the red-green-refactor programming concept. The ultimate goal of stubbing out a class is to ensure that all of it's methods fail at runtime before the actual implementation of those methods.
For the Calculator class, try the below sample code and see how it goes
Hope this helps!
using System;
public class Calculator { public double Result; public Calculator (double result) { Result = result; } public void Initialize() { }
public void Add(double val)
{
}
}