Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial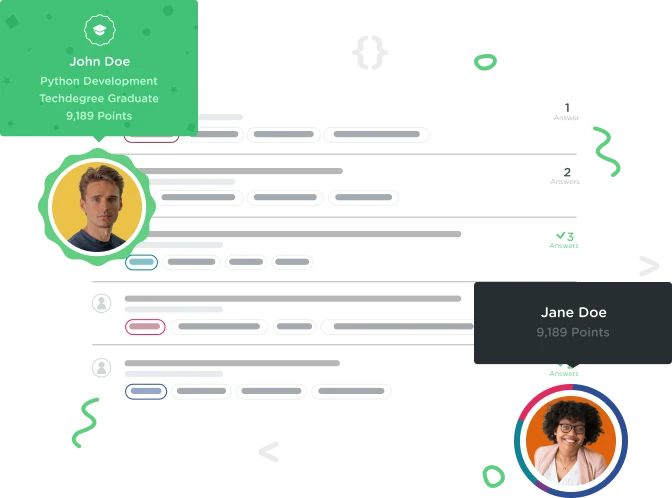

Devanshi verma
1,307 Pointswhat mistake am I making?
help
var secret = prompt("What is the secret password?");
while(secret !=="sesame"){
document.write("You know the secret password. Welcome.");
secret+=1;
}
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="app.js"></script>
</body>
</html>
3 Answers
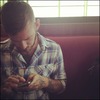
Erik McClintock
45,783 PointsDevanshi,
What we want to happen with this code is for there to be an initial prompt to the user to enter a secret password, and until they enter it correctly, we want them to continue to be prompted for it.
First, we have our variable secret
set up to store the value that gets entered into our prompt. Next, we create a while
loop that checks to see if the user has entered the correct password, and if they have not, will show the prompt again. Once the user clears the while
loop, i.e. once they have successfully entered the password, the loop will break and our code will move on to the next line, which will be when we finally write to the document that they know the secret password.
Let's take a look at this in code:
// here we set up our variable and set it equal to the prompt that asks for the password
var secret = prompt( "What is the secret password?" );
// here we create our "while" loop, that says "while secret does not equal 'sesame', prompt the user to enter the password again"
while( secret !== "sesame" ) {
// by setting the variable secret equal to our prompt again inside the "while" loop, we successfully loop the user into our prompt until they enter the correct password!
secret = prompt("What is the secret password?");
}
// once the user has entered the correct password into the prompt, the loop will pass, and we will reach this code
document.write("You know the secret password. Welcome.");
Hope this helps to clear things up!
Erik

Nikhil Deorkar
Courses Plus Student 1,695 PointsI don't think that you are using a while loop correctly. Loops in general are for repeating tasks, not for trying to find out user input and respond to it. And while they can on specific occasions, I think that instead, you need to use an if statement there instead. Observe:
var password = prompt("What is the password?")
if (password != "sesame") {
document.write("You know the secret password. Welcome.");
} else { Do whatever }
Also, if that doesn't do what you wanted it to do, you might have wanted to make it so the password is equal to "sesame". In that case, change the not equal to(!=) to an equal to(===). Hope this helped!

Jacob Mishkin
23,118 Pointsyou almost have it! you just need to make a few changes.
- create an empty var named secret outside of the while loop.
var secret;
- create a while loop that has the prompt inside the while loop. You want the loop to continue unit the user enters sesame.
while(){
secret = prompt('what is the secret password');//this will make the prompt appear unit the condition is false
}
- add the condition to the while loop
while(secret !== 'sesame')
- remove the counter in the while loop.
I hope this helps.