Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial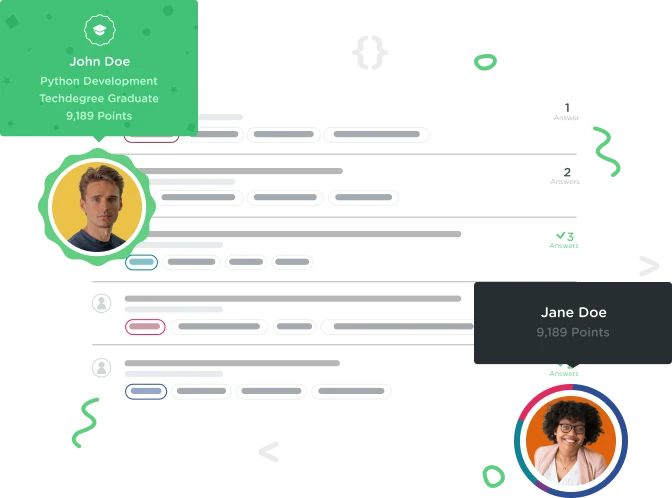
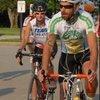
Gerald Tinney
15,454 Pointswhat purpose does the empty Console.WriteLine serve t the end of the if else statement?
it runs without error either way...
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
Console.WriteLine("please enter your pimp name or \"quit\" to exit here: ");
string input = Console.ReadLine();
if (input == "quit")
{
Console.WriteLine("Goodbye.");
}
else
{
Console.WriteLine ("You entered " + input + ".");
}
Console.WriteLine();
}
}
}
2 Answers

andren
28,558 PointsWith the way your code is written it does indeed not serve any purpose beyond creating an empty line, however that is because of the changes you have done to the challenge code, the challenge code starts out like this:
input = Console.ReadLine();
if (input == "quit")
{
string output = "Goodbye.";
}
else
{
string output = "You entered " + input + ".";
}
Console.WriteLine(output);
And while your solution is completely valid, it's not the solution Treehouse expected, this is the expected solution:
string input = Console.ReadLine();
string output;
if (input == "quit")
{
output = "Goodbye.";
}
else
{
output = "You entered " + input + ".";
}
Console.WriteLine(output);
With that solution the last Console.WriteLine call is necessary for the code to work.
As you get further into programming you'll learn that there are almost never only one way to solve a particular problem in programming, there can be hundreds of ways to accomplish the same task.
Since you ended up with a solution slightly different from the one that was intended, some of the placeholder code ended up being redundant, that's all there is to it.

Anjali Pasupathy
28,883 PointsIn the version of the code you created, you can include Console.WriteLine() if you want to put some space between this execution of Program and whatever comes next in the console.
In the original code, Console.WriteLine was used to print out the output.
Console.WriteLine(output);
While your solution to the challenge works with or without the Console.WriteLine() at the end, it isn't the only solution. Another solution would be to declare an output variable of type string before the if-else statement, and to remove "string" from the instantiation of output. In this solution, Console.WriteLine(output) after the if-else statement couldn't be removed without changing the intended function of the code.
class Program
{
static void Main()
{
string input = Console.ReadLine();
string output; // DECLARE output VARIABLE
if (input == "quit")
{
output = "Goodbye."; // REMOVE "string" TO ASSIGN TO PREVIOUSLY DECLARED output VARIABLE
}
else
{
output = "You entered " + input + "."; // REMOVE "string" TO ASSIGN TO PREVIOUSLY DECLARED output VARIABLE
}
Console.WriteLine(output); // THIS LINE CANNOT BE REMOVED IN THIS SOLUTION
}
}
I hope this helps!