Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial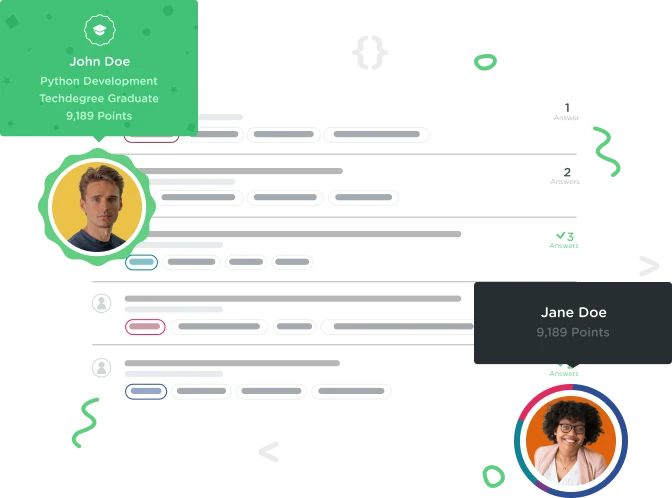

Luke Wunderlich
759 PointsWhat should I do?
I'm learning splitting and joining lists in Python. The first task asks me to split the available variable by the semicolons:
available = "banana split;hot fudge;cherry;malted;black and white"
sundaes = available.split(";")
That part worked fine. Second task was putting in the menu variable:
available = "banana split;hot fudge;cherry;malted;black and white"
sundaes = available.split(";")
menu = "Our available flavors are: {}."
I just can't figure out the third task which is asking me to join the available list with a comma and a space, then formatting it to the menu variable.
available = "banana split;hot fudge;cherry;malted;black and white"
sundaes = available.split(";")
menu = "Our available flavors are: {}."
sundaes.join(", ")
menu.format(sundaes)
Once I run the code, it tells me that task 1 is no longer passing. Any help?
1 Answer
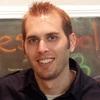
Rick Buffington
8,146 PointsThe problem you are facing starts with your join command. You need to call the join command on a string, not the array.
", ".join(sundaes)
So in essence, they want you to substitute that in the output somehow (I don't know the exact challenge so I can't tell you for sure, but this should get you in the right direction).
print menu.format(", ".join(sundaes))
Hopefully that helps.

Luke Wunderlich
759 PointsAfter I add your first line of code, it gives me the same warning. "Task 1 is no longer passing." And if I add both of them, it gives the same warning, and if I add only the second line, it gives the same warning also.
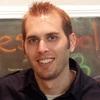
Rick Buffington
8,146 Points1) Create a variable named sundaes that uses split
sundaes = available.split(";")
2) Assign a new menu variable to the string "Our available flavors are: {}."
menu = "Our available flavors are {}."
3) Using .format() on the menu variable, join the sundaes items with ", " and assign them to a new menu variable.
menu = menu.format(", ".join(sundaes))
That should complete all of the tasks. They want you to overwrite by assignment the menu variable using the format function and the join functions.
Luke Wunderlich
759 PointsLuke Wunderlich
759 PointsThank you so much, Rick!