Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial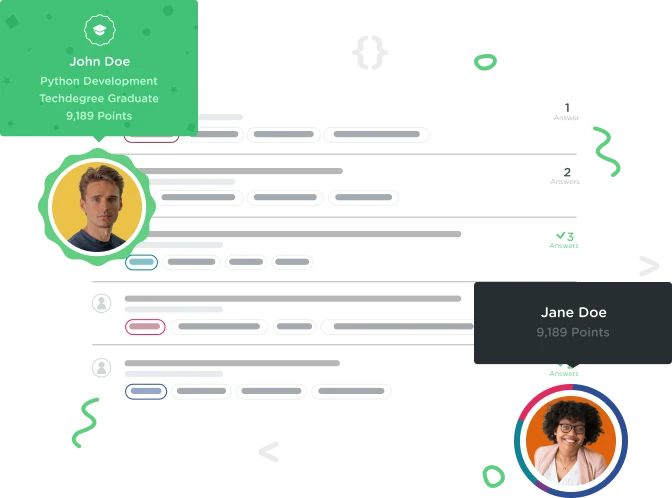

Elisha Redmond
385 PointsWhat should this code say ?
User = input ('what is your favorite color ')
favorite_color = "Red"
User = "favorite_color"
print(" The color", user , "is a great color")
1 Answer
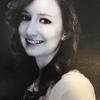
Megan Amendola
Treehouse TeacherHi, Elisha! You should be setting the user's input to the variable favorite_color
. Then you print the variable with the statement "The color __ is a great color!"
Let me walk you through your code to show you what's happening:
User = input ('what is your favorite color ')
First, you set the input to the variable User
instead of favorite_color
. The input message should also be "What is your favorite color? ".
favorite_color = "Red"
Then you set the favorite_color
variable to the string 'Red'
. favorite_color
should be equal to the input so it will then equal whatever the user inputs in as they're favorite color. It should not be only one color.
User = "favorite_color"
Here, you then overwrote the User
variable to now equal the string 'favorite_color'
. This variable now holds a string.
print(" The color", user , "is a great color")
Lastly, your print message will not run because you don't have a variable called user
only User
. Even if it did, it would print out " The color favorite_color is a great color". This message should print out whatever color the user inputs and should look something like this: "The color Purple is a great color!".
Punctuation and correct spelling matter in code challenges too, so make sure your input message and print out message match what the challenge is asking you for.
The code should look something like this where you capture the user's input in a variable called favorite_color
and then print it out with the message.
favorite_color = input("What is your favorite color? ")
print('The color {} is a great color!'.format(favorite_color))
Elisha Redmond
385 PointsElisha Redmond
385 PointsThis really helps thanks