Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial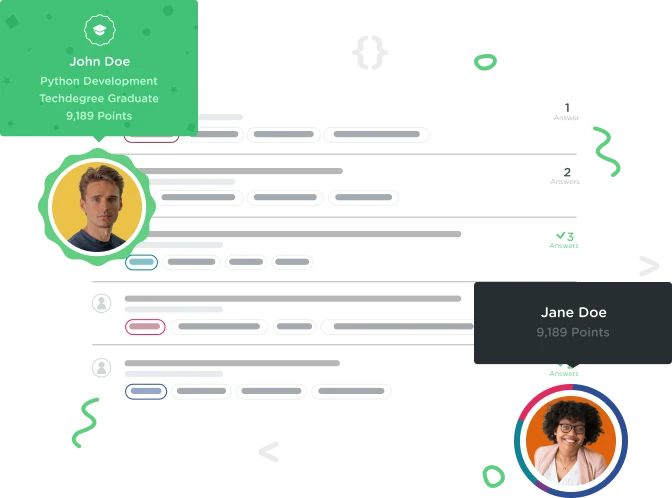

hend elsisi
621 Pointswhat the benefit of convenience initializer if do same job with initialize the class members with default values
i cant understand the difference between the convenience initializer and assigning default values in the class declaration
2 Answers
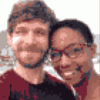
Chris Nash
Courses Plus Student 1,402 PointsConvenience initializers give you incredible control over how something is initialized. Check out my example... it uses cats! Everyone loves cats.
Example: A program that creates four cats, and outputs their color and age. Depending on what parameters are provided at the moment of creation, the correct convenience initializer is used.
- Otto - color: "white" // age: 10
- Muffins - color: NOT GIVEN // age: 5
- Bruno - color: "red" -// age: NOT GIVEN
- Pico - NOTHING GIVEN
class Cat {
var color: String
var age: Int
//If both parameters color and age are given:
init (color: String, age: Int) {
self.color = color
self.age = age
}
//If only age is given:
convenience init (age: Int) {
self.init(color: "black", age: age)
}
//if only color is given:
convenience init (color: String) {
self.init(color: color, age: 1)
}
//if nothing given
convenience init () {
self.init(color: "black", age: 1)
}
}
//create Otto:
var Otto = Cat(color: "white", age: 10)
println("Otto is \(Otto.color) and \(Otto.age) year(s) old!")
//create Muffins:
var Muffins = Cat(age: 5)
println("Muffins is \(Muffins.color) and \(Muffins.age) year(s) old!")
//create Bruno:
var Bruno = Cat(color: "red")
println("Bruno is \(Bruno.color) and \(Bruno.age) year(s) old!")
//create Pico:
var Pico = Cat()
println("Pico is \(Pico.color) and \(Pico.age) year(s) old!")
CONSOLE OUTPUT:
Otto is white and 10 year(s) old!
Muffins is black and 5 year(s) old!
Bruno is red and 1 year(s) old!
Pico is black and 1 year(s) old!

Jonas Östlund
3,012 PointsThis can be answered a bit in the course but i will try to give a relative easy answer:
If we have a class "car" that has three variables: color, wheels and doors:
class Car {
var wheels: Int
var doors: Int
var color: String
}
If we would set these variables as default values then every car would look the exactly same. But if we can do a initializer then we can customize every car:
class Car {
var wheels: Int
var doors: Int
var color: String
init(wheels: Int, doors: Int, color: String) {
self.wheels = wheels
self.doors = doors
self.color = color
}
}
A convenience initializer could also be added to easy create a common car:
class Car {
var wheels: Int
var doors: Int
var color: String
init(wheels: Int, doors: Int, color: String) {
self.wheels = wheels
self.doors = doors
self.color = color
}
convenience init (color: String) {
self.wheels = 4
self.doors = 2
self.color = color
}
}
This could also be done by default values to the three variables but if some of the values would need to be manipulated by a function or method Xcode would complain about trying to access a method that is not accessible so initialize the values in a initializer creates more possibilities to manipulate values
class Car {
var wheels: Int
var doors: Int
var color: String
init(wheels: Int, doors: Int, color: String) {
self.wheels = wheels
self.doors = doors
self.color = color
}
convenience init (color: String) {
self.wheels = 4
self.doors = 2
self.color = createColor(color)
}
func createColor(color: String) -> UIColor {
return UIColor(red: 1, green: 1, blue: 1, alpha: 1)
}
}

Tim Luk
5,140 PointsI don't think your code for the convenience init is not right.
This should be the right one. convenience init(color: String){ self.init(wheels: 4, doors: 2, color: color)
Lawrence Yeo
3,236 PointsLawrence Yeo
3,236 PointsGreat clarification on that question. Thanks, Chris!
kavi ram
183 Pointskavi ram
183 PointsThis can be accomplished by providing default defination in init() method