Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial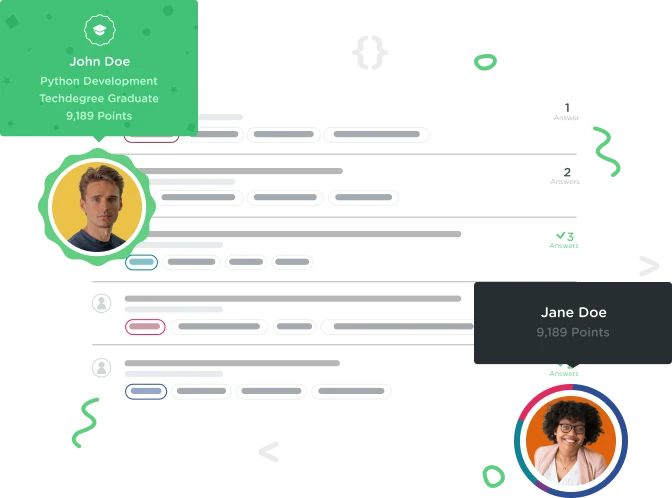
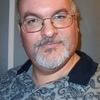
Richard Haven
528 PointsWhat the hell is " Bummer! Try again!" ?!
That is a very counterproductive error message, especially in a teaching context.
What did I do wrong? Am I missing an import? You run the code through a Python compiler, so you have the error message.
At worst, say, "You're doing something wrong, but I cannot figure out what. Maybe re-watch the previous video"
import datetime
from peewee import *
from flask.ext.restful import Resource
DATABASE = SqliteDatabase('recipes.db')
class Recipe(Model):
name = CharField()
created_at = DateTimeField(default=datetime.datetime.now)
class Meta:
database = DATABASE
# TODO: Ingredient model
# name - string (e.g. "carrots")
# description - string (e.g. "chopped")
# quantity - decimal (e.g. ".25")
# measurement_type - string (e.g. "cups")
# recipe - foreign key
class Ingredient(Model):
name = CharField()
description = CharField()
quantity = DecimalField()
measurement_type = CharField()
recipe = ForeignKeyField(Recipe)
class Meta:
database = DATABASE
class IngredientList(Resource):
list = []
def get(index):
return self.list[index].toString()
class Ingredient(Resource):
def get:
return "Foo"
def toString:
return get
from flask.ext.restful import Resource
import models
3 Answers

Ryan S
27,276 PointsHi Richard,
There are quite a few issues with your code so a concise error message might not be possible. I'll try to clear things up.
The first major issue is that your resources should be defined in "resources/ingredients.py" (the other tab in the code challenge), not in "models.py". This is actually the first reason why you aren't getting any useful error messages, because you haven't written any code in the file.
Secondly, all class methods must take a "self" argument, but the "get" method for Ingredient
will require an additional "id" argument.
And lastly, there is quite a bit that needs to be cleared up regarding the methods you've defined so it might be easier to start over. For example, you've defined an unnecessary empty list in IngredientList
and are using the list indexing incorrectly. Also, you are attempting to call a method (toString) that is defined in the other resource. The "toString" method is unnecessary and not asked for in the challenge.
Each get()
method only needs to return a string.
from flask.ext.restful import Resource
import models
class IngredientList(Resource):
def get(self):
return "Foo"
class Ingredient(Resource):
def get(self, id):
return "Foo"
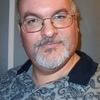
Richard Haven
528 PointsTry what again?
I figured out what I did wrong, but this is a horrible teaching technique
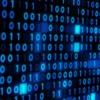
Alexander Davison
65,469 PointsActually, one of the most important skills in programming is to find and fix bugs in your code. So no, I don't agree with you. Tracking down bugs are hard, but with practice, you can find them easier.

mattweil
123 PointsBummer means that you got it wrong. And that you should try again.