Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial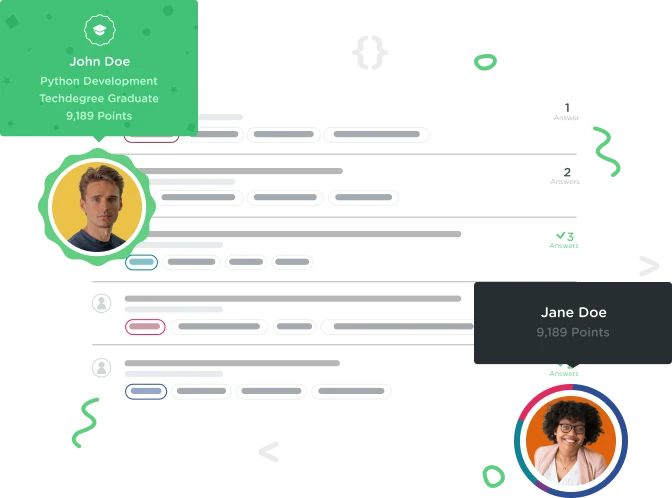

dlpuxdzztg
8,243 PointsWhat to do from here?
So i'm doing the code challenge for this lesson and I set up the member variable, i'm not sure what to put in the 'if' statement though.
Help is appreciated!
public class TeacherAssistant {
private static String mFirstName;
public static String validatedFieldName(String fieldName) {
// These things should be verified:
// 1. Member fields must start with an 'm'
// 2. The second letter in the field name must be uppercased to ensure camel-casing
// NOTE: To check if something is not equal use the != symbol. eg: 3 != 4
mFirstName = fieldName;
if (mFirstName) {
throw new IllegalArgumentException();
}
return fieldName;
}
}
2 Answers
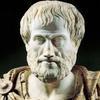
Florian Tönjes
Full Stack JavaScript Techdegree Graduate 50,856 PointsSup Diego,
like the challenge says:
1 . Check if the first character of the String that is coming in is 'm'.
- Use the .getCharAt() method to get the first character of 'fieldName' and then use the ' != ' operator to check if it doesn't equal the character 'm'.
2 . Check if the second character is uppercase.
- Use .getCharAt() method to get the second character of 'fieldName' this time. Use the '!' operator and the Character.isUpperCase() method to check if it is not uppercase.
3 . Combine the two boolean expressions.
- Use the ' || ' operator to combine both expressions. If the first or the second is true throw the IllegalArgumentException.
Also, you don't need the 'mFirstName' variable that you declared. Also because it is a static class variable you wouldn't want to prefix it with 'm' and go with 'firstName' instead.
Kind Regards, Florian

Uriah Nevins
1,121 PointsTo start, you need to check if the first letter in the variable is an "m". So in the If statement, you can use a charAt function. After that, you need to make sure that the second letter is uppercase. Here is the code that I have;
public class TeacherAssistant {
public static String validatedFieldName(String fieldName) {
// These things should be verified:
// 1. Member fields must start with an 'm'
// 2. The second letter in the field name must be uppercased to ensure camel-casing
// NOTE: To check if something is not equal use the != symbol. eg: 3 != 4
if (fieldName.charAt(0) != 'm' || !(Character.isUpperCase(secondLetter(fieldName)))) {
throw new IllegalArgumentException("Wrong Syntax.");
}
return fieldName;
}
public static char secondLetter(String fieldName){
return fieldName.charAt(1);
}
}
dlpuxdzztg
8,243 Pointsdlpuxdzztg
8,243 PointsOh...I thought it was asking me to create a member variable...huge difference!
Thanks!