Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial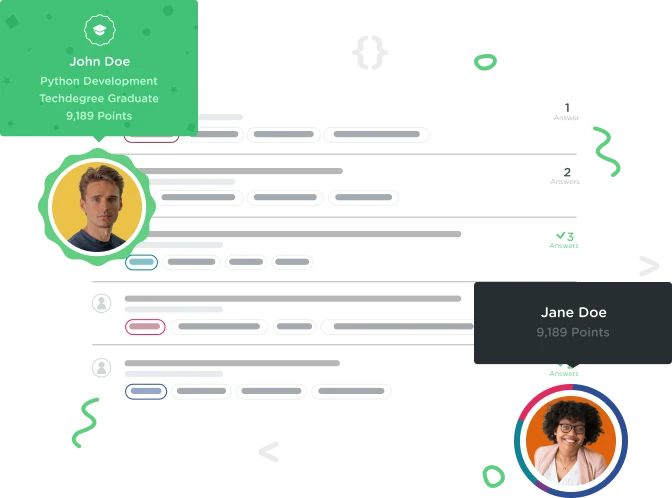

Amit Ahuja
706 PointsWhat to use for return statement
I know I'm missing a return statement but I can't figure out what to use for it to return as a (Double, Double)
// Enter your code below
func getTowerCoordinates(location: String) -> (Double, Double) {
switch location {
case "Eiffel Tower": (48.8582, 2.2945)
case "Great Pyramid": (29.9792, 31.1344)
case "Sydney Opera House": (33.8587, 151.2140)
default: (0,0)
}
}
1 Answer

Greg Kaleka
39,021 PointsHi Amit,
Sorry - my initial answer was wrong. I wasn't paying close enough attention :).
Your switch statement is actually not doing anything at all. It checks the value of location, and then just "says" a tuple. You need to do one of two things:
- Return the tuple directly
- Store the tuple in a variable, and then return the variable.
Here's how that would look:
func getTowerCoordinates(location: String) -> (Double, Double) {
switch location {
case "Eiffel Tower": return (48.8582, 2.2945)
case "Great Pyramid": return (29.9792, 31.1344)
case "Sydney Opera House": return (33.8587, 151.2140)
default: return (0,0)
}
}
OR
func getTowerCoordinates(location: String) -> (Double, Double) {
var coordinates: (Double, Double)
switch location {
case "Eiffel Tower": coordinates = (48.8582, 2.2945)
case "Great Pyramid": coordinates = (29.9792, 31.1344)
case "Sydney Opera House": coordinates = (33.8587, 151.2140)
default: coordinates = (0,0)
}
return coordinates
}
Amit Ahuja
706 PointsAmit Ahuja
706 PointsHi Greg,
I actually tried that before and kept getting an error of not being able to convert a String into (Double, Double). This is what I currently have. Is there something wrong with the formatting?
Thanks
Greg Kaleka
39,021 PointsGreg Kaleka
39,021 PointsHey sorry Amit - completely whiffed on my first answer. I've edited my answer above.