Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial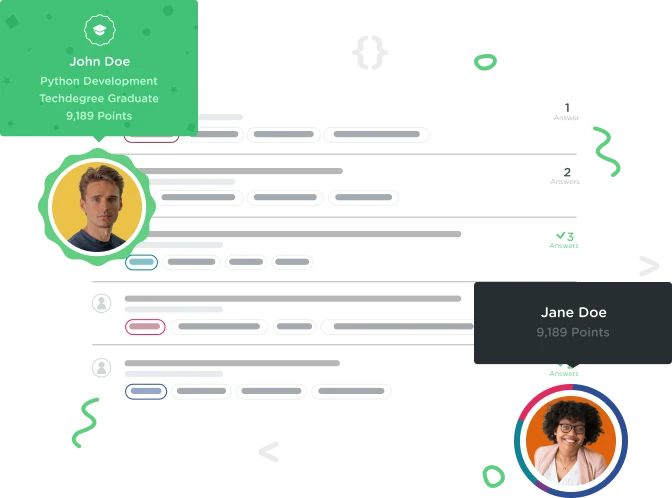

Ilhyun Jo
6,288 PointsWhat was the point of using guard to check item quantity. Wouldn't a if statement be more fitting?
I thought guard statements were used to unwrap optionals so the first guard statement was understandable but the second one seemed unreasonable since there was no optional to unwrap.
guard item.quantity > quantity else { throw VendingMachineError.OutOfStock }
if item.quantity < quantity { throw VendingMachineError.OutOfStock }
2 Answers

Greg Kaleka
39,021 PointsThe advantage of guard is that if the criteria isn't met, the code will leave the current scope. If you use if, the code will run through the inside of the if statement, and then continue with the rest of the code in the current scope, which you might not want. Here's an example.
func giveOutItems {
guard item.quantity > quantity else {
throw VendingMachineError.OutOfStock
} // if we go into the guard statement, we won't continue after this line
charge(item, quantity) // we don't want to do this if there's an error!
}
If we'd used an if statement, the error would have been thrown, and then the vending machine would have charged the customer for the full price anyway! There are other ways around this without using guard, but guard is simpler in many cases.
Hope this helps!
-Greg

Paul Foltz
9,952 PointsThe top-voted answer is simply untrue. A throw
statement will immediately transfer program control out of the current method. Any statements following the throw
statement will not be executed. To achieve the same logic in the video using an if-statement, you can invert the condition:
if item.quantity <= 0 {
throw VendingMachineError.OutOfStock
}
Ilhyun Jo
6,288 PointsIlhyun Jo
6,288 PointsThanks your explanation made things clear!
Mert Kahraman
12,118 PointsMert Kahraman
12,118 PointsThanks!
James Estrada
Full Stack JavaScript Techdegree Student 25,866 PointsJames Estrada
Full Stack JavaScript Techdegree Student 25,866 PointsWhat's the difference if I use an if-else statement?