Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial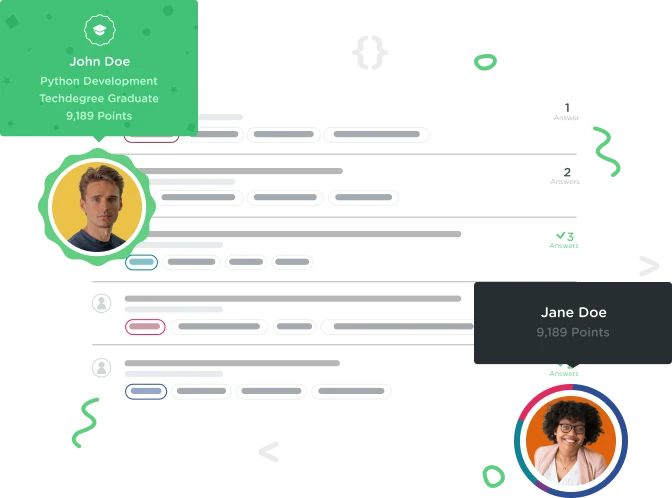
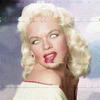
norman cole
2,756 PointsWhat wrong with my code
I need you to create a new function for me. This one will be named sillycase and it'll take a single string as an argument. sillycase should return the same string but the first half should be lowercased and the second half should be uppercased. For example, with the string "Treehouse", sillycase would return "treeHOUSE". Don't worry about rounding your halves, but remember that indexes should be integers. You'll want to use the int() function or integer division, //.
# creat new function named sillycase/ Takes single argument.
treehouse = "treehouse"
def sillycase(treehouse):
half = round(len(string)/2)
return string[:half].lower() + string[half:].upper()
#return string first half lowercased and the second half uppercased
# indexes should be integers
#use the int()
1 Answer

Kent Γ svang
18,823 PointsOkay, so there's a couple of things..
# creat new function named sillycase/ Takes single argument.
""" this first variable is okay """
treehouse = "treehouse"
""" here you have passed threehouse between the parenthesis, which is a-okay, but remember that this doesn't actually
pass anything to the function, it is just a placeholder name for when you CALL the function, but here we declare it,
so you can call it anything you like """
def sillycase(treehouse):
""" this won't work. you round the length of a string divided by two, but in essence there to many unknown here to
get any calculation. """
half = round(len(string)/2)
""" Since for variable 'half' won't work, this will not work either. """
return string[:half].lower() + string[half:].upper()
#return string first half lowercased and the second half uppercased
# indexes should be integers
#use the int()
I will try to guide you through how I would do it, and maybe that will clarify a thing or two.
# First I declare the function that takes one argument. Since the argument passed in will be a string,
# I'll choose to call it, 'a_string'
def sillycase(a_string):
# Now I want to get the length of the string passed into my function :
length_of_string = len(a_string)
# Now since I have the length of the string, I can also get half the length :
half_the_length = length_of_string / 2
# Now, since the number could be odd, I have to make sure it is an integer, and not a double.
# I can do this passing in the int
half_the_length_as_int = int(halv_the_length)
# Now I can using the number stored in 'half_the_length_as_int' to get the first and second half of the string
first_half = a_string[:half_the_length_as_int]
second_half = a_string[half_the_length_as_int]
# fixing the upper and lower case
first_half = first_half.lower()
second_half = second_half.upper()
# Joining them togheter
silly_case = first_half + second_half
# than return it
return silly_case
""" Of course, I have tried to dum it down and really emphasize the steps taken, but the code could actually be
written like this also """
def sillycase(a_string):
half_point = int(len(a_string) / 2)
first_half_lower = a_string[:half_point].lower()
second_half_upper = a_string[half_point:].upper()
return first_half_lower + second_half_upper
Hope that this helped somewhat.