Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial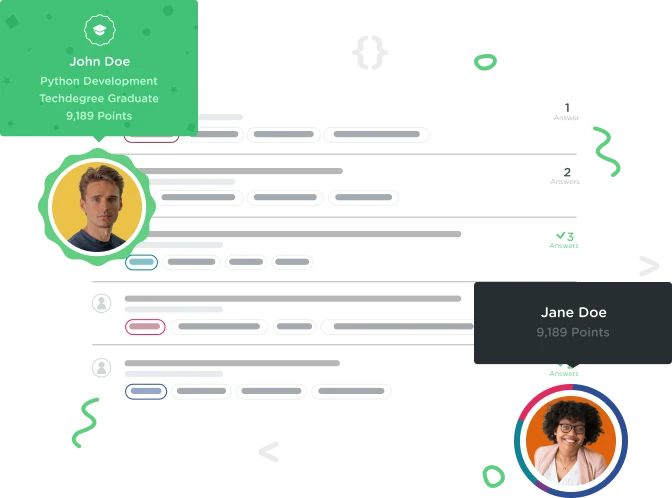

Vlatko .
2,526 PointsWhat's a more efficient way of listing animation images?
In the lesson each image of the animation is listed in the array, like so:
self.backgroundImageView.animationIamges = @[[UIImage imageName:@"01"], [UIImage imageName:@"02"], [UIImage imageName:@"03"]];
There's 60 images for the animation, which means 60 lines of code. There definitely has to be an easier way to achieve this. If so, what is it?
Also side note, what's the formatting label for Objective C on this forum?
1 Answer
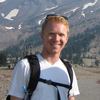
Rutger Farry
10,722 PointsI was going to say that it would probably be pretty easy to throw together a for-loop to do that using the addObject:
and stringWithFormat:
methods, but the animationImages property is not a mutable array. Therefore, a for loop wouldn't work unless you made a temporary mutable array and then instantiated animationImages
with that array, like so
// make a temporary, mutable array
NSMutableArray *tempArray = [NSMutableArray array];
for (int i = 1; i < 61; i++) {
if (i < 10) {
// create a string with the image's name
NSString *imageName = [NSString stringWithFormat:@"CB0000%d", i];
// add the image to the array
[tempArray addObject:[UIImage imageNamed:imageName]];
}
else {
NSString *imageName = [NSString stringWithFormat:@"CB000%d", i];
[tempArray addObject:[UIImage imageNamed:imageName]];
}
}
self.backgroundImageView.animationImages = [NSArray arrayWithArray:tempArray];
Note: The if/else statement is to make the correct imageName. If I is less than 10, there is an extra zero proceeding the number. Another note: This is probably terribly memory inefficient and you shouldn't do it. Just collapse your code or figure out a better way.
Mike Lange
794 PointsMike Lange
794 PointsThis was my first thought also when I watched the video - "why isn't the instructor mentioning a more efficient way to code the images" - since obviously we want to be efficient with our coding and not repeat code unnecessarily.
I'd like to understand the impact on device memory using a loop technique using a mutable array or if it should just plain be avoided.
If this technique is to be avoided and is seriously poor memory management, a quick mention in the training video would have been good, but since it wasn't mentioned, can we get an official answer on this?