Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial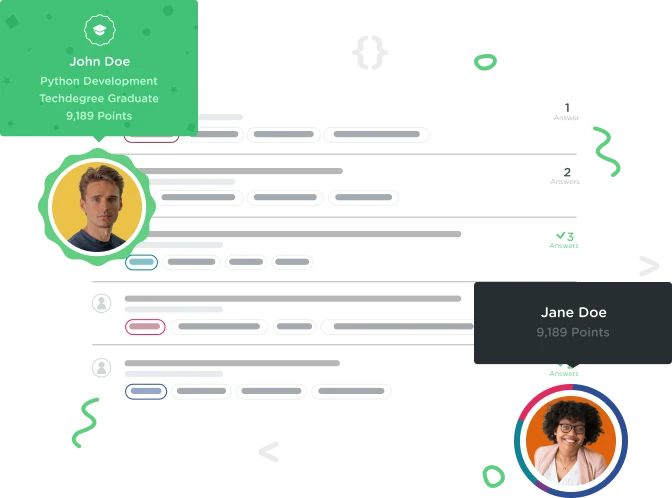

Jacob Kesey
1,715 PointsWhat's causing my code to open the print preview screen on Chrome?
var questions = [
['How many states are in the United States?', 50],
['How many continents are there?', 7],
['How many legs does an insect have?', 6]
];
var correctAnswers = 0;
var question;
var answer;
var response;
var correct = [];
var wrong = [];
function buildList(arr) {
var listHTML = '<ol>';
for (var i = 0; i< arr.length; i +=1 ) {
listHTML += '<li>' + arr[i] + '</li>';
}
listHTML += '<ol>';
return listHTML;
}
for (var i = 0; i < questions.length; i += 1) {
question = questions[i][0];
answer = questions[i][1];
response = prompt(question);
response = parseInt(response);
if (response === answer) {
correctAnswers += 1;
correct.push(question);
} else {
wrong.push(question);
}
}
var html = "You got " + correctAnswers + " question(s) right."
html += '<h2>You got these questions correct:</h2>';
html += buildList(correct);
html += '<h2>You got these questions wrong:</h2>';
html += buildList(wrong);
print(html);
2 Answers

Umesh Ravji
42,386 PointsHi Jacob, you are calling the print method window.print()
, hence you are getting the print dialog coming up. If you write your own function named print
inside your js file, that will replace the existing print
function, removing this problem :)

Jacob Kesey
1,715 PointsThanks Umesh, that fixed the problem. That also raises another question - is naming a function after another function a common practice? Would it be better to name it something like htmlPrint or onScreenPrint, etc... ?

Umesh Ravji
42,386 PointsYou could name it something different, but there are other ways to deal with this issue. Take a look at this example, using a self invoking anonymous function.
(function() {
function print() {
console.log('Print function called..');
}
document.addEventListener('click', print);
})();
You can click anywhere on the page, and it will call the print method, but since it is wrapped within a function, it won't replace the print method defined on the window object. Not a useful example, but I hope it illustrates the point.