Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial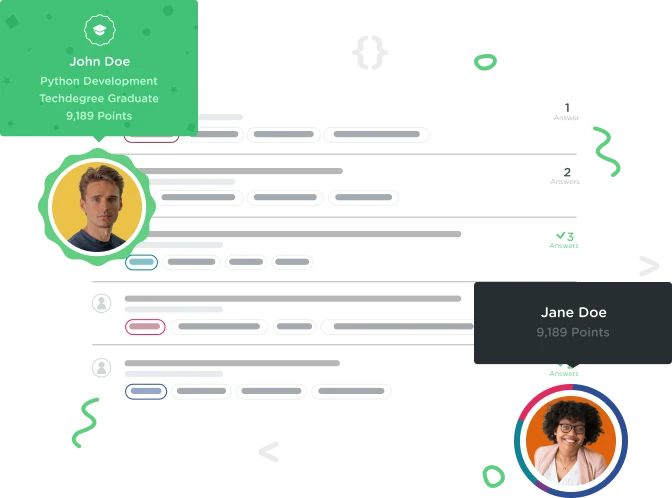

Jackson Monk
4,527 PointsWhat's going on?
The below code is acting strange. All the words you type come out as worth one point. I am confident it has to do with the loop that iterates through all the letters. Even when you print out the value of letterCounter directly, it prints out 1 for however many letters there are. There is also a missing closing parenthese that I can't find, but that is not as big an issue. Help is appreciated, thank you.
<!DOCTYPE html>
<html>
<head>
<title>Page Title</title>
</head>
<body>
<center>
<h1>Scrabble Words</h1>
<h3>How to Play: Enter a word or words and press enter and you will see how much each word you typed is worth and what your highest scoring word is</h3>
<hr/>
<input type = text>
<button>Enter</button>
<hr/>
</center>
<ul></ul>
<script>
// defining HTML variables
let input = document.getElementsByTagName('input')[0];
let button = document.getElementsByTagName('button')[0];
let ul;
let li;
let text;
let text2;
let li2;
// defining what the button does
button.addEventListener('click', () => {
let input = document.getElementsByTagName('input')[0];
// defining javascript variables
let words;
let letters;
let written;
let emparr = [];
let conarr = [];
let wnarr = [];
let letterCounter = 0;
// making an array of letters
let v = ['a', 'b', 'c', 'd', 'e', 'f', 'g', 'h', 'i', 'j', 'k', 'l', 'm', 'n', 'o', 'p', 'q', 'r', 's', 't', 'u', 'v', 'w', 'x', 'y', 'z'];
written = input.value;
// creating an empty array
letters = [];
// splitting the sentence into words
words = written.split(" ");
// splitting words into letters
for (let i = 0; i < words.length; i += 1) {
letters += [words[i].split("")];
}
// giving each letter of each word a scrabble letter value
for (let j = 0; j < letters.length; j += 1) {
if (letters[j].toLowerCase() === v[0] || v[4] || v[8] || v[11] || v[13] || v[14] || v[17] || v[18] || v[19] || v[20]) {
letterCounter += 1;
}
else if (letters[j].toLowerCase() === v[3] || v[6]) {
letterCounter += 2;
}
else if (letters[j].toLowerCase() === v[2] || v[12] || v[15]) {
letterCounter += 3;
}
else if (letters[j].toLowerCase() === v[5] || v[7] || v[21] || v[22] || v[24]) {
letterCounter += 4;
}
else if (letters[j].toLowerCase() === v[10]) {
letterCounter += 5;
}
else if (letters[j].toLowerCase() === v[9] || v[23]) {
letterCounter += 8;
}
else if (letters[j].toLowerCase() === v[16] || v[25]) {
letterCounter += 10;
}
}
// using the empty array to store the letterCounter value of each word in the form of an array in
emparr += [letterCounter];
conarr.push(letterCounter);
letterCounter = 0;
}
for (let h = 0; h < words.length; h += 1) {
text = document.createTextNode("Your word " + words[h] + " is worth " + emparr[h] + " point(s)");
li = document.createElement('li');
let ul = document.getElementsByTagName('ul')[0];
li.appendChild(text);
ul.appendChild(li);
text = undefined;
li = undefined;
ul = undefined;
input.value = "";
}
for (let q = 0; q < words.length; q += 1) {
wnarr += [words[q], conarr[q]];
}
let sorter = conarr.sort(function(a, b) {
return b - a;
});
for (let o = 0; o < wnarr.length; o += 1) {
if (sorter[0] === wnarr[o][1]) {
text2 = document.createTextNode("Your highest scoring word is " + wnarr[o][0] + " with a value of " + wnarr[o][1]);
li2 = document.createElement('li');
li2.appendChild(text2);
ul.appendChild(li2);
}
}
});
// coloring elements
button.style.color = 'white';
button.style.backgroundColor = 'blue';
button.style.border = 'blue';
// turning the textbox gray when you scroll over it
input.addEventListener('mouseover', () => {
input.style.backgroundColor = 'lightgray';
});
input.addEventListener('mouseout', () => {
input.style.backgroundColor = 'white';
});
</script>
</body>
</html>
'''
2 Answers
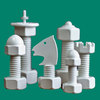
Steven Parker
229,771 PointsWhen combining comparison expressions with logic operators, each side of the logic operator needs to be a complete comparison.
For example:
letters[j].toLowerCase() === v[3] || v[6] // this expression is always "true"
letters[j].toLowerCase() === v[3] || letters[j].toLowerCase() === v[6] // do THIS instead

Jackson Monk
4,527 PointsOkay, it should be better now
Steven Parker
229,771 PointsSteven Parker
229,771 PointsWhen posting code, use the instructions for code formatting in the Markdown Cheatsheet pop-up below the "Add an Answer" area.
 Or watch this video on code formatting.