Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial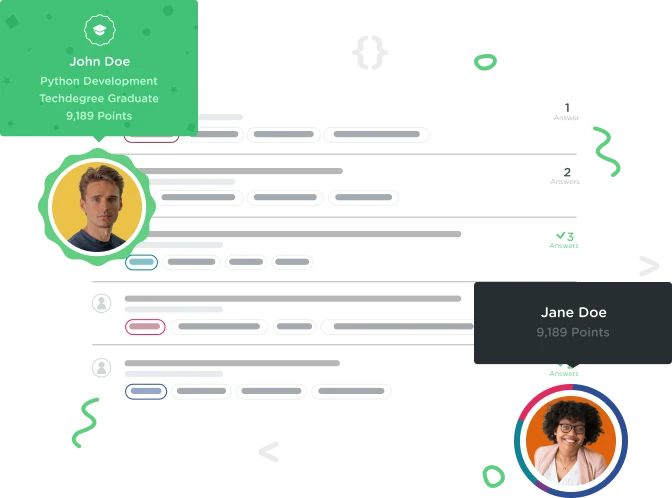

mohammed saleh
210 Pointswhats going on here guys, what am I possibly doing wrong. I am trying to return the year but keeps giving me error.
whats going on here guys, what am I possibly doing wrong. I am trying to return the year but keeps giving me error.
function getYear (){
var year = new Date().getFullYear();
}
return year;
<!DOCTYPE HTML>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>JavaScript Basics</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
4 Answers
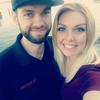
Bryan Reed
11,747 PointsYou're closing your function before you return it. Your return line needs to be in the function brackets, not outside of them
function getYear(){
var year = new Date().getFullYear();
return year
}
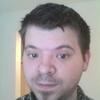
Aaron Loften
12,864 PointsBryan is right...well, he missed a semi-colon. Even though the interpreter might correct it for you, you should probably assume that it wont and finish it. :)
The way a function return works, in short, is you define a function, you call a function, and if needed return a result.
function someTest() {
//do something
var result = true;
return result;
}
//call the function - you can alert, or console.log() the function to see the return value
someTest();
We do this so we can do things like...get a result from a function, get a value, or maybe just in general knwoing that a function completed. A practical use of the function return is as follows...
if(someTest() == true) {
//do something
} else {
//do something else
}
Returning outside of a function would not make sense, even if it worked, because the script wouldnt know which thing to return a result for.
Its also worth mentioning that a return should be toward the end of a function. When a return fires off, it ends the function. So placing a return in the right spot can sometimes be key to producing a great function. Like as follows...
function someTest(someValue) {
if(someValue == 1) {
return "The answer is 1";
}
if(someValue == 2) {
return "The answer is 2";
}
return "Unexpected result :(";
}
//call the function with a value passed through
someTest(2);
So, to fix your problem...
function getYear(){
var year = new Date().getFullYear();
return year;
}

Rich Donnellan
Treehouse Moderator 27,708 PointsFixed your code formatting. From the Markdown Cheatsheet:
If you specify the language after the first set of backticks, that'll help us with syntax highlighting.

mohammed saleh
210 Pointstop lad bryan great lad. u are a legen, dnt knw y I didnt pick up on this, silly me.

mohammed saleh
210 PointsThanl you guys, your answers really helped.
Rich Donnellan
Treehouse Moderator 27,708 PointsRich Donnellan
Treehouse Moderator 27,708 PointsFixed your code formatting. From the Markdown Cheatsheet: