Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial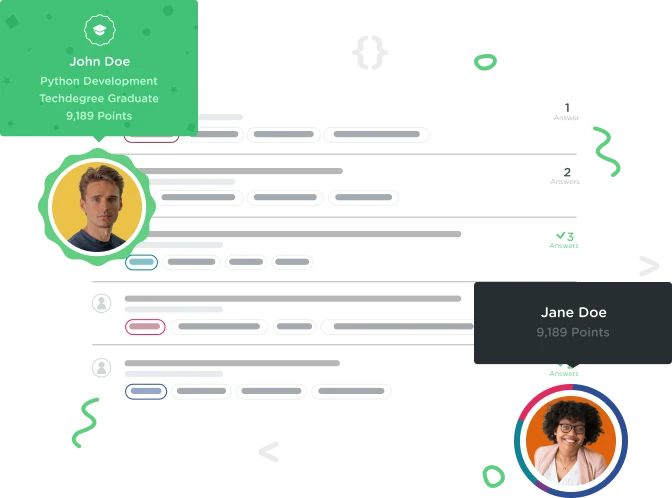
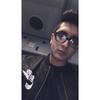
Hasseeb Hussain
2,154 PointsWhat's gone wrong here?
I'm not sure what's gone wrong here.
class Point {
var x: Int
var y: Int
init(x: Int, y: Int) {
self.x = x
self.y = y
}
}
class Machine {
var location: Point
init() {
self.location = Point(x: 0, y: 0)
}
func move(_ direction: String) {
print("Do nothing! I am a machine!")
}
}
// Enter your code below
class Robot: Machine {
override func move(direction: String) {
switch direction {
case "Up": location.y += 1
case "Down": location.y -= 1
case "Left": location.x -= 1
case "Right": location.x += 1
default: break
}
}
}
1 Answer

andren
28,558 PointsWhen your code does not compile it can often be a good idea to take a look at the compiler error that Swift generates, they are often more helpful than you might think. You can see them by clicking on the "Preview" button after trying to run your code.
The error generated for your code is this:
swift_lint.swift:33:17: error: argument names for method 'move(direction:)' do not match those of overridden method 'move'
override func move(direction: String) {
^
_
Which is essentially just telling you that you don't use the exact same argument names for the overridden move
method as the original one does. Which is a requirement when overriding a method.
Specifically you have forgotten to include _
as an external parameter name. If you add that like this:
class Point {
var x: Int
var y: Int
init(x: Int, y: Int) {
self.x = x
self.y = y
}
}
class Machine {
var location: Point
init() {
self.location = Point(x: 0, y: 0)
}
func move(_ direction: String) {
print("Do nothing! I am a machine!")
}
}
// Enter your code below
class Robot: Machine {
override func move(_ direction: String) {
switch direction {
case "Up": location.y += 1
case "Down": location.y -= 1
case "Left": location.x -= 1
case "Right": location.x += 1
default: break
}
}
}
Then your code will work.
Hasseeb Hussain
2,154 PointsHasseeb Hussain
2,154 PointsSilly mistake! Thanks.