Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial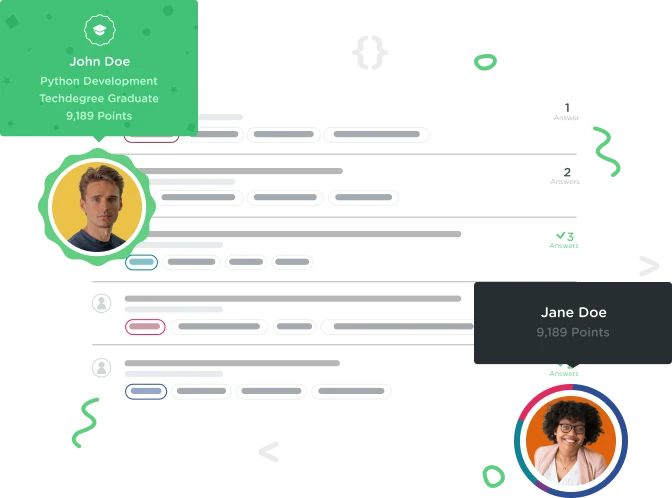

ishay vilroel
1,279 PointsWhat's is wrong?
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
while(true)
{
Console.Write("Enter the number of times to print \"Yay!\": ");
var entry = Console.ReadLine();
try
{
var NumberOfTimes = int.Parse(entry);
while(NumberOfTimes>0)
{
Console.WriteLine("Yay!");
NumberOfTimes--;
}
}
catch (FormatException)//ArgumentNullException
{
Console.WriteLine("You must enter a whole number.");
continue;
}
break;
}
}
}
}
6 Answers

Lewis Cowles
74,902 PointsYou need to name your exceptions as a parameter
catch (FormatException)//ArgumentNullException
Should be
catch (FormatException e)//ArgumentNullException
Also the commenting out like you have done is not really good practice; use version control solutions such as Git, that way you don't have to comment out; you can roll-back to a previous commit, or better still utilize good Git workflow and keep all changes in a branch you can delete if it leads to a dead end (kinda like evolution)

ishay vilroel
1,279 Pointsi did what you told me but it still does not work...

Lewis Cowles
74,902 PointsJust a tip in future, include the error that "it" shows, as "it" will always show an error, or have a way to view errors if "it" does not work, as there is no great way of me finding out what "it" is, or what is wrong without this information...
Also the code runs via workspaces via mcs Program.cs && mono Program.exe
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
while(true)
{
Console.Write("Enter the number of times to print \"Yay!\": ");
var entry = Console.ReadLine();
try
{
var NumberOfTimes = int.Parse(entry);
while(NumberOfTimes>0)
{
Console.WriteLine("Yay!");
NumberOfTimes--;
}
}
catch (FormatException e)//ArgumentNullException
{
Console.WriteLine("You must enter a whole number.");
continue;
}
break;
}
}
}
}
It also works without the namespace, however creating the Exception as I have advised without using it will generate a compiler warning
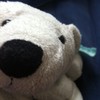
bothxp
16,510 PointsHi Lewis,
The code in your response doesn't appear to pass the task in the challenge. Did it work for you?

Lewis Cowles
74,902 PointsI passed the challenge the day it was released, I never had these problems
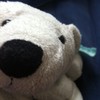
bothxp
16,510 PointsHi ishay,
Now I'm not 100% sure on this ... but it appears that the issue is your 'continue' in your catch statement.
It looks as if the code that is testing your answer is not expecting the outer loop that you created. So if it enters the catch and then reaches the continue then the test process is not expecting to have to provide another number, so you get the null error.
I think it is just expecting to print out the "You must enter a whole number." message and then end ... not loop round to ask for another number.
So taking out your outer loop and removing the break & continue you get:
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
Console.Write("Enter the number of times to print \"Yay!\": ");
var entry = Console.ReadLine();
try
{
var NumberOfTimes = int.Parse(entry);
while(NumberOfTimes>0)
{
Console.WriteLine("Yay!");
NumberOfTimes--;
}
}
catch (FormatException)//ArgumentNullException
{
Console.WriteLine("You must enter a whole number.");
}
}
}
}
which I believe does pass.
I think that the way in which you answered is probably the way I would have done it if I was expecting a real person to be using it. Looping around again and asking for another number seems completely logical, I just don't think it was what the challenge is expecting you to do and so the code that is running the test isn't set up for it ....... I think that's what's going on.
I hope that helps

Lewis Cowles
74,902 PointsRight I don't like doing this, as I like to fix the immediate problem and continue, but here is the code I just passed the challenge with (completely ignoring your original input)
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
Console.Write("Enter the number of times to print \"Yay!\": ");
var response = Console.ReadLine();
var times = 0;
try {
times = int.Parse(response);
}
catch( FormatException ) {
Console.Write("You must enter a whole number");
}
if( times < 0 ) {
Console.Write("You must enter a positive number.");
}
for( var i=0; i<times; i++ ) {
Console.Write("Yay!");
}
}
}
}
- lacks your outer while loop (the program exits as soon as it is done)
- uses a for loop instead of a while loop (bothxp's while loop is fine also I just didn't use it)
- as in the course I made sure I gave the value of the input a default parsed value (in case of errors) of 0
- also includes the final check to complete the entire challenge (not just .2)

ishay vilroel
1,279 PointsThe continue was the problem !
ishay vilroel
1,279 Pointsishay vilroel
1,279 Pointssomething with--> System.ArgumentNullException: Argument cannot be null. Parameter name: String. See output for stack trace.