Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial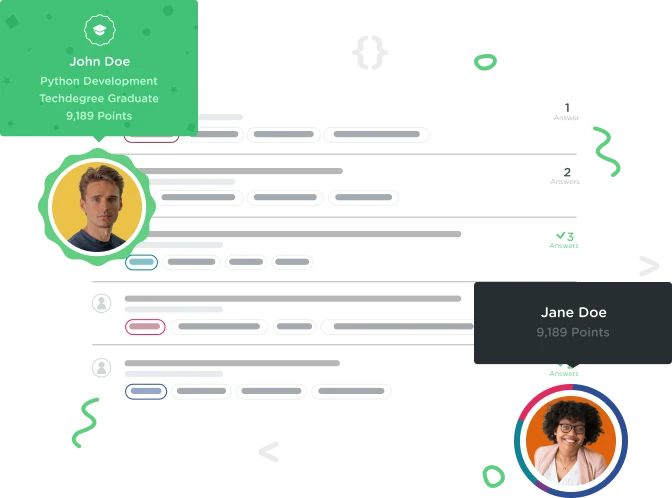

SZE SZE XU
9,759 Pointswhats math.floor, math.random the video mentioned?
whats math.floor, math.random?
Complete the code below to call the function and pass the value 12 to it: function getRandom( upper ) { return Math.floor(Math.random() * upper) + 1; }
?????
2 Answers
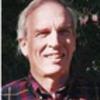
jcorum
71,830 PointsSZE, Java has many, many built-in classes. One of those is the Math class. The Math class has a bunch of methods. If you go to https://docs.oracle.com/javase/7/docs/api/, click on java.lang in the top left panel, then click Math in the list of classes in the bottom left panel, you will see a list of all of the Math class's properties and methods. Among the methods you will find floor() and random().
Note that Java is case-sensitive, so you cannot write math.random() and have it compile. You must write Math.random(). Also, note that because Math's methods are static, you call them like this: Math.random(), unlike other classes, where you have to create an object first and then call methods on the object.
Math.floor(), Math.sqrt(), Math.abs(), etc.
versus Random:
Random r = new Random();
int x = r.nextInt();
For static methods like Math's it is ClassName.methodName. For non-static method's like Random's it's objectName.methodName
As the API will show you, you can use Math's methods to get the square root of a number (sqrt), you can round down (floor), you can get the absolute value (abs), etc.
So Math.abs(-1) would return 1, Math.sqrt(9) would return 3, etc.
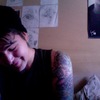
Chris Gonzalez
2,365 PointsI know this was explained in great detail by previous poster but I also want to just foot note that Math.floor is a native method of the javascript built in library that basically just floors the decimal place so you always have a whole number like 12.3 will return 12. open your browser inspector and run a line with Math.floor(12.9); .
it will return 12
Math.ceil is the total opposite, if a decimal place exist on any given number it will bring it to the next number
Math.ceil(12.1) will return 13. Math.ceil(12) will return 12.
So you are basically building a random number generator. The reason they have you floor the Math.Random() number is because it will return a decimal times your upper.
upper is the variable you made up with your highest number range if i remember clearly, The reason you add one is because there is a very very insanely small chance that your Math.random(); call will actually roll a 0.0 which isn't very helpful if you are making dice.. there is no 0 side on a die.
I hope this helped none the less. Just keep at it and it and constantly echo if you are lost or confused.

SZE SZE XU
9,759 Pointsvery clear, thanks