Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial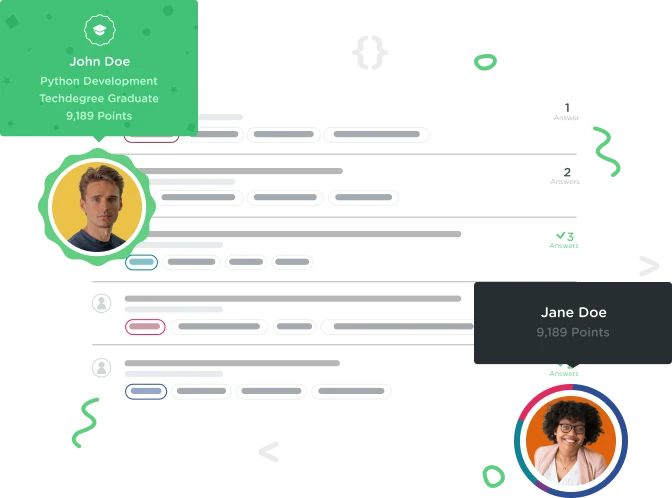
1 Answer

Simon Coates
28,694 PointsIf it isn't handled immediately, it jumps up the call stack, until it finds code to handle it (a try/catch for the appropriate exception). I don't think you need to know the terminology, just how exceptions behave.
If methodOne called methodTwo, and methodTwo calls methodThree, then an exception in methodThree will bubble up to methodTwo unless it's handled. If methodTwo doesn't handle it, it will bubble up to methodOne. So you have options on where to handle the exception (methodTwo or methodOne), or whether you want to handle it in multiple places (catch, attempt partial recovery and rethrow it so that the calling method is notified).
case 1: CRASHES WHOLE APP
class Main {
public static void main(String[] args) {
methodOne();
System.out.println("I will not run");
}
public static void methodOne() {
methodTwo();
System.out.println("I will not run");
}
public static void methodTwo() {
methodThree();
System.out.println("I will not run");
}
public static void methodThree() {
String shortString = "a";
shortString.charAt(45); //causes an exception
}
}
case2: Handle exception at a higher level
class Main {
public static void main(String[] args) {
methodOne();
System.out.println("I will run");
}
public static void methodOne() {
try {
methodTwo();
System.out.println("I will not run");
} catch(StringIndexOutOfBoundsException e){
System.out.println("Run code to recover from exception");
}
}
public static void methodTwo() {
methodThree();
System.out.println("I will not run");
}
public static void methodThree() {
String shortString = "a";
shortString.charAt(45); //causes an exception
}
}
Case 3: Handle, throw and Rehandle
class Main {
public static void main(String[] args) {
try {
methodOne();
System.out.println("I will not run");
} catch(Exception e){
System.out.println("Run code to recover from exception");
}
}
public static void methodOne() throws Exception {
try {
methodTwo();
System.out.println("I will not run");
} catch(StringIndexOutOfBoundsException e){
System.out.println("Run code to recover from exception and rethrow");
throw e;
}
}
public static void methodTwo() {
methodThree();
System.out.println("I will not run");
}
public static void methodThree() {
String shortString = "a";
shortString.charAt(45); //causes an exception
}
}