Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial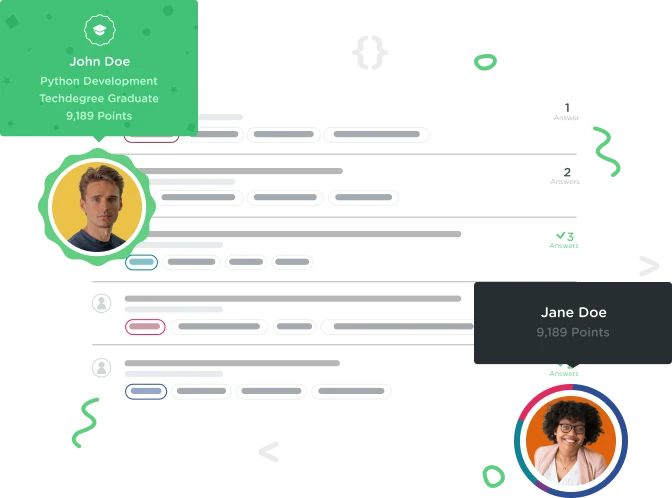

peterson st gourdain
931 Pointswhats missing or I'm I using the else if wrong
help
var isAdmin = true;
var isStudent = false;
if ('isAdmin = ('true')') {
} else if (alert("Welcome administrator")); {
}
<!DOCTYPE HTML>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>JavaScript Basics</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
1 Answer
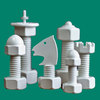
Steven Parker
230,688 PointsHere's a few hints:
- statements and expressions should not be enclosed in quotes
- boolean values (like true) should not be enclosed in quotes
- a single equal sign ("=") is an assignment operator, a comparison operator is two equal signs ("==")
- the parentheses following an "if" should contain a comparison expression
- conditional code goes between the braces that come after the conditional expression
- there should not be a semicolon between an "if" condition and the brace of the code block
Jonas Westerholm
10,754 PointsJonas Westerholm
10,754 PointsOk, lets have a look at the first line.
if ('isAdmin = ('true')')
isAdmin is a variable and should thus not be used with ' '-marks around it. When using ' ' or " " javascript will think it's a string of characters. The same goes for true. True and false are boolean values (think 1 or 0) and should be used without quotation marks.
= One equal sign (=) sets a variable, while two or three (== or ===) checks if the variables on both sides of the comparator are equal.
The else if syntax is wrong, and it's not needed to use it at all in this case.
This would be the correct solution for this problem
Should you want to use the else if and else in javascript the solution could look something like this
*** For the complete list of issues, see Stevens hints ***