Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial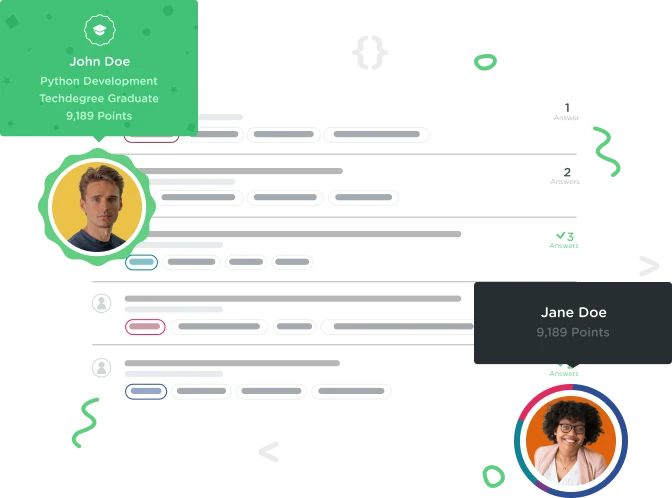
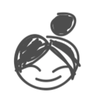
Linlin Huang
2,413 PointsWhat's the advantage of using a Do..While loop?
I don't see what the advantage of using a Do...While loop is over using just a conditional with a While loop.
To achieve the same results as the video (in Javascript Loop, Arrays, and Objects: Do... While Loops), I can write this as well, and it's the shorter than the code written in the video. So, why would it be better to use a Do...While loop than doing something like this?
var randomNumber = getRandomNumber(10);
var guess;
var guessCount = 0;
function getRandomNumber( upper ) {
var num = Math.floor(Math.random() * upper) + 1;
return num;
}
guess = parseInt( prompt("Guess a number between 1 and 10") )
guessCount++
while (guess !== randomNumber) {
guess = parseInt( prompt("That was wrong! Guess another number between 1 and 10") )
guessCount++
}
document.write("<p> You guessed the number, which was " + randomNumber + ".</p>")
document.write("<p> It took you " + guessCount + " guesses.</p>")
2 Answers
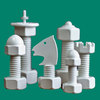
Steven Parker
231,533 Points
A "do...while
" loop runs the code before testing the condition.
This guarantees that the code will be executed at least once, even if the condition is already false.
On the other hand, a conventional "while
" loop tests the condition first, and if it is false the code is not executed at all.
Any potential "advantage" to one method over the other will depend on the specific situation.
Update: Someone asked nearly this same question just two days later!
It got some similar (but much more verbose) answers.

Jhuanderson Macias
1,870 PointsThis may be a bit ridiculous but lets say that you wants to bake 5 cookies, but if friends come over you ll cook more cookies:
do{
bake 5 cookies;
} while (thereAreFriends);
no matter what, you will bake 5 cookies(even if no friends come over), but if friends do come over then you will bake 5 more cookies per friend Of course you can get this by using a while do loop as well, but it just depends on the situation. The video was prob just getting you used to do..while loops.