Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial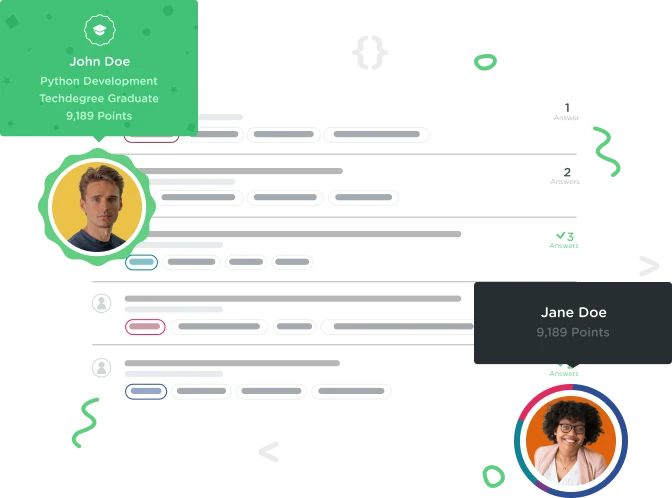

Howie F
1,710 PointsWhat's the answer?
I can't figure out why this isn't working. I think I'm not understanding the for. . .in construct.
For example, with the statement:
for month in months {
what is "month" exactly? does it need to be declared as a variable? The explanation in the video wasn't very helpful/precise enough.
let months = [1, 2, 3]
for month in months {
if months[month] == 1 {
println("January")
} else if months[month] == 2 {
println("February")
} else {
println("March")
}
}
3 Answers

Hayes McCardell II
643 Pointsmonths is an array of integers 1, 2, and 3.
month is one of those integers. So you don't need to access it like a typical array, since 'month' is standing in for the value of that index.
let months = [1, 2, 3]
for month in months {
if month == 1 {
println("January")
} else if month == 2 {
println("February")
} else {
println("March")
}
}
This may help out: https://developer.apple.com/library/ios/documentation/Swift/Conceptual/Swift_Programming_Language/ControlFlow.html

Howie F
1,710 PointsThanks! I think I get it now. So to make sure I understand how this works.
- month is a variable that refers to the value of the array at whatever index
- the for. . in loop goes sequentially through the values of the array (e.g., months[0], months[1], months[2]. . .) until it goes through the entire array
Does that also mean month is declared implicitly? In this and the other samples of for. . .in loops, the variable after the "for" is never declared.
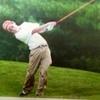
kjvswift93
13,515 PointsWhen it comes to 'month', when you write "for month in months", you are essentially declaring a new constant named month of type Int.
let month: Int
Now, month can be used to reference any of the Int values within the array. Note that month cannot be assigned to any of the integers in the array, only compared (==).