Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial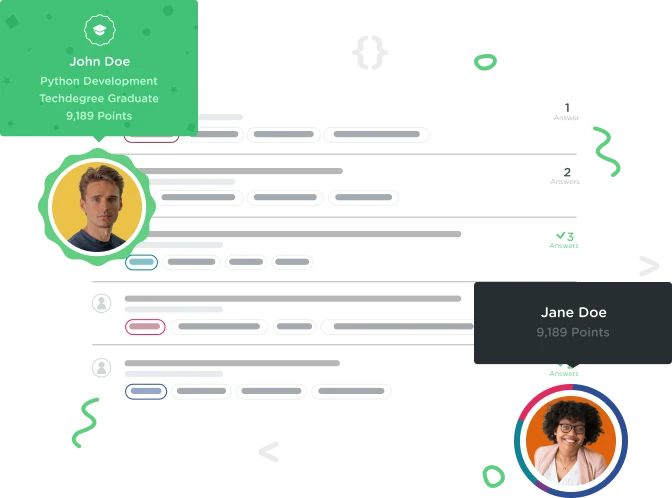

Ben Dyson
Courses Plus Student 6,715 PointsWhats the correct way to define a function
I've been defining function like this:
function sayHello()
{
}
However in the video Jim does it like:
var sayHello = function()
{
}
Which is the correct way?
3 Answers

Jeremy Hayden
1,740 PointsThey both work. In your first example you are declaring a function.
In your second example you're declaring a variable that is referencing an anonymous function
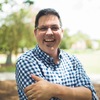
Dave McFarland
Treehouse TeacherAs the others here have mentioned both methods create functions. I would recommend that you put the first brace on the same line as the function declaration like this:
function myFunction() {
}
This is called the "1 true brace style" and is the more common way to write braces in JavaScript. You're examples use the K&R style which is commonly used in C and C++. You can put the initial brace on its own line below function
but it's not as common.

James Barnett
39,199 PointsThe short answer is they are both valid JavaScript.
For the long answer, that requires going into more depth about how functions and hoisting work in JavaScript.
These topics are covered in greater depth in the Javascript Foundations course.
Andrea Mangano
Courses Plus Student 3,567 PointsAndrea Mangano
Courses Plus Student 3,567 PointsFUNCTION DECLARATION or NAMED FUNCTION
A function declaration is not executed immediately. It creates a function that you can call later in your code. In order to call the function you must give it a name, so these are know as named functions. A function created with a function declaration can be called before it has even been declared.
ANONYMOUS FUNCTION
An anonymous function is a function that is not given a name when it is created. While you can't reference an anonymous function after it has been defined, you can reference it at the time that it is defined. If you create an anonymous function and assign it to a variable immediately when the function is defined then the function can be referenced via that variable in the same way as it could be if you gave the function that name when you created it.
What is the difference between an NAMED FUNCTION and ANONYMOUS FUNCTION?
The difference is that when you assign an anonymous function to a variable the variable only points to the function from that point in your code onward and you can assign a different value to the variable at any time to replace the function. Named functions have global scope and so are less flexible in how you can use them.