Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial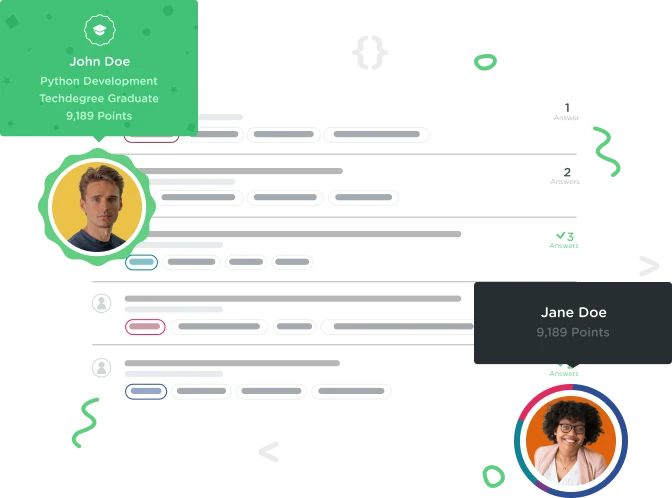

jeancruz
Courses Plus Student 3,123 PointsWhat's the correct way to handle bad PKs?
I tried putting everything in a single line:
article = get_object_or_404(articles, pk=pk)
But it didn't work either.
from django.shortcuts import render, get_object_or_404
from .models import Article, Writer
def article_list(request):
articles = Article.objects.all()
return render(request, 'articles/article_list.html', {'articles': articles})
def writer_detail(request, pk):
writer = Writer.objects.get(pk=pk)
return render(request, 'articles/writer_detail.html', {'writer': writer})
def article_detail(request, pk):
try:
article = Article.objects.get(pk=pk)
except:
article = get_object_or_404(article, pk=pk)
return render(request, 'articles/article_detail.html', {'article': article})
from django.conf.urls import url
from . import views
urlpatterns = [
url(r'writer/(?P<pk>\d+)/$', views.writer_detail),
url(r'article/(?P<pk>\d+)/$', views.article_detail),
url(r'', views.article_list),
]
1 Answer
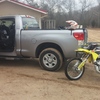
Ryan Ruscett
23,309 PointsA PK is like an ID that you can query results on based on some index within the model or database for intensive purposes.
pk=3 implies an ID of 3. So i can query results and get just the 3rd ID or index.
Now say my table only has 2 columns and I say PK=3. It will fail with things like list integer out of range. The best way to handle these is to catch the object class exceptions or since models are objects. you could try and catch ObjectDoesNotExist and a whole slew of other ones.. You can of course check for specifics in the documentation but unless you have a really large performance intensive project. No real need to worry about catching specific errors. Just catch em all.