Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial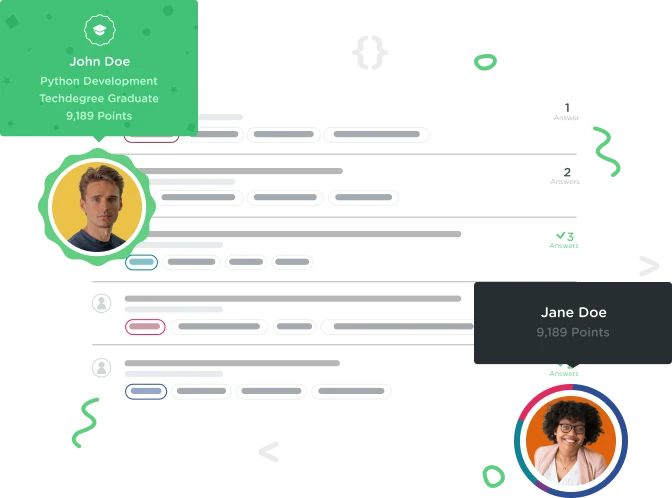

daniel zaragoza
1,843 PointsWhat's the difference?
I've been playing around with the code and i noticed that both pieces of code below give the same output (Unless im goin crazy ). Also, why did the instructor choose to write the code like the first example below wouldn't the second example be faster?? Thanks in advance
Example 1:
var giveNum1 = prompt(" Type starting number ");
var bottomNum = parseInt(giveNum1);
var giveNum2= prompt(' Pick second number ');
var topNum= parseInt(giveNum2);
var randomNum = Math.floor( Math.random() * (topNum - bottomNum + 1)) + bottomNum;
alert(" You picked " + randomNum);
Example 2;
var num1= prompt(" Type starting number ");
var num2 = prompt(" Type second number ");
var randomNum = Math.floor( Math.random() * (parseInt(num2) - parseInt(num1) + 1 )) + parseInt(num1);
alert(randomNum);
3 Answers
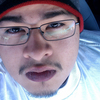
Chyno Deluxe
16,936 PointsThere is no difference other than readability. When you use variables, you are replacing the variable name with the value given to it.
Your example one is exactly the same as your example 2. You are simply removing the extra lines of code and variables.

Jason Anello
Courses Plus Student 94,610 PointsHi Daniel,
Dave likely wrote it as example 1 to make it easier to understand and read. He has to write the code from the perspective of teaching the subject and not necessarily how we would write the code if we were building a website or application.
For me personally, I think example 1 is easier to read. I don't like seeing all those parseInt calls within the random number calculation. That expression is already long enough without them being in there. But it really comes down to personal preference.
Faster in what way? Faster to type or faster in performance?
Example 2 might be slightly faster to type but I wouldn't make any coding decisions based on how long it's going to take to type.
In terms of performance, example 2 might be slightly slower because you have 3 calls to parseInt instead of 2 calls like in example 1. I didn't test this so don't hold me to it. :)
Any performance difference between these 2 is likely to be very insignificant.
As a general rule, I wouldn't sacrifice code readability for a small gain in performance. It's not worth it.
One simplification that I would be comfortable making with example 1 is this:
var bottomNum = parseInt(prompt(" Type starting number "));
var topNum= parseInt(prompt(' Pick second number '));
var randomNum = Math.floor( Math.random() * (topNum - bottomNum + 1)) + bottomNum;
alert(" You picked " + randomNum);
You can take the result of the prompt call and pass it directly into the parseInt function. I don't think this sacrifices readability and it does get rid of a few variables.
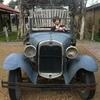
Brendan Moran
14,052 PointsThis is exactly how I did it, and this is my first time learning this! Patting my noob self on the back. :)

Muhammad Nur
811 PointsHow about this? This seems to work for me.
var bottomNumber = parseInt(prompt("bottomnumber"));
var topNumber = parseInt(prompt("topnumber"));
console.log(bottomNumber, topNumber)
var randomNumber = Math.floor(Math.random() * topNumber) + bottomNumber;
console.log(randomNumber);