Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial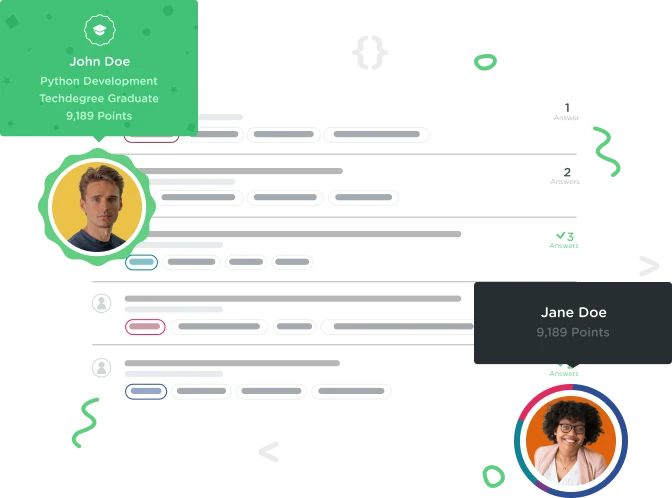

Ronny Kibet
47,409 PointsWhats the difference between a struct and a class in swift?
What's the difference between struct and class.
2 Answers

Stone Preston
42,016 Pointsthe main difference is that structs are value types and classes are reference types.
When you make a copy of a value type, it copies all the data from the thing you are copying into the new variable. They are 2 seperate things and changing one does not affect the other
When you make a copy of a reference type, the new variable refers to the same memory location as the thing you are copying. This means that changing one will change the other since they both refer to the same memory location
Here is an article from apple comparing structs and classes
this post on SO has a nice example. The top answer is below:
<Here's an example with a class. Note how when the name is changed, the instance referenced by both variables is updated. Bob is now Sue, everywhere that Bob was ever referenced.
class SomeClass {
var name: String
init(name: String) {
self.name = name
}
}
var aClass = SomeClass(name: "Bob")
var bClass = aClass // aClass and bClass now reference the same instance!
bClass.name = "Sue"
println(aClass.name) // "Sue"
println(bClass.name) // "Sue"
And now with a struct we see that the values are copied and each variable keeps it's own set of values. When we set the name to Sue, the Bob struct in aStruct does not get changed.
struct SomeStruct {
var name: String
init(name: String) {
self.name = name
}
}
var aStruct = SomeStruct(name: "Bob")
var bStruct = aStruct // aStruct and bStruct are two structs with the same value!
bStruct.name = "Sue"
println(aStruct.name) // "Bob"
println(bStruct.name) // "Sue"
So for representing a stateful complex entity, a class is awesome. But for values that are simply a measurement or bits of related data, a struct makes more sense so that you can easily copy them around and calculate with them or modify the values without fear of side effects.

Ronny Kibet
47,409 PointsThank you Stone. That covers it. :) Well done.

tytyty
4,974 PointsQuestion. To be clear...In the Class example Stone said that aClass and bClass now reference the same instance at 'var bClass = aClass'. Is the instance 'name'? Also why are var and init both using 'name'?
Sebastian King
4,834 PointsSebastian King
4,834 Pointsspicy answer thanks :)